Enhancing Your React Development Workflow with React Developer Tools
React Developer Tools is a browser extension that allows developers to inspect and debug React components in their applications. It provides a set of tools that make it easier to understand and manipulate the component hierarchy, inspect component properties and state, and optimize performance. React Developer Tools is available for Chrome, Firefox, and Edge browsers, and it is an essential tool for any React developer.
One of the main benefits of using React Developer Tools is that it provides a visual representation of the component hierarchy, also known as the React Component Tree. This allows developers to easily navigate through the different components in their application and understand how they are structured. It also helps in identifying any issues or bugs in the component hierarchy, making it easier to debug and fix them.
Key Takeaways
- React Developer Tools is a browser extension that helps developers debug and optimize React applications.
- Installing React Developer Tools is easy and can be done through the Chrome or Firefox extension stores.
- The React Component Tree allows developers to visualize the hierarchy of components in their application.
- Inspecting component properties and state can help developers identify and fix bugs in their code.
- React Developer Tools can also be used to optimize performance by identifying and addressing performance bottlenecks.
Installing React Developer Tools
Installing React Developer Tools is a straightforward process. Here is a step-by-step guide on how to install it:
1. Open your preferred browser (Chrome, Firefox, or Edge).
2. Go to the browser’s extension store (Chrome Web Store, Firefox Add-ons, or Microsoft Edge Add-ons).
3. Search for “React Developer Tools” in the extension store.
4. Click on the “Add to Chrome/Firefox/Edge” button.
5. Confirm the installation by clicking on “Add extension” or similar.
6. Once installed, you should see the React Developer Tools icon in your browser’s toolbar.
Alternatively, you can also install React Developer Tools from the official website (https://reactjs.org/blog/2019/08/15/new-react-devtools.html). Simply download the extension file for your browser and follow the installation instructions provided.
Exploring the React Component Tree
The React Component Tree is a hierarchical structure that represents the relationship between different components in a React application. It starts with the root component and branches out to child components, forming a tree-like structure.
With React Developer Tools, you can easily explore the React Component Tree of your application. Simply open the developer tools panel in your browser and navigate to the “Components” tab. Here, you will see a visual representation of the component hierarchy.
You can expand and collapse different components to view their child components. This helps in understanding how the components are nested and how they interact with each other. You can also inspect the props and state of each component, which we will discuss in the next section.
Inspecting React Component Properties and State
Property/State | Description | Type | Default Value |
---|---|---|---|
props | The properties passed to the component | Object | {} |
state | The internal state of the component | Object | {} |
componentDidMount() | Method called after the component is mounted | Function | – |
componentDidUpdate(prevProps, prevState) | Method called after the component is updated | Function | – |
shouldComponentUpdate(nextProps, nextState) | Method that determines if the component should update | Function | true |
React components have properties (props) and state, which determine their behavior and appearance. React Developer Tools allows you to inspect these props and state values, making it easier to understand how a component is functioning.
To inspect the props and state of a component, simply select the component in the React Component Tree view. In the right-hand panel, you will see two tabs: “Props” and “State”. Clicking on these tabs will display the corresponding values for the selected component.
The “Props” tab shows the props passed to the component, including their names and values. This is useful for understanding how data is being passed down from parent components to child components.
The “State” tab shows the current state of the component. It displays all the state variables defined in the component, along with their current values. This is helpful for debugging and understanding how the component’s state changes over time.
Debugging React Components with React Developer Tools
React Developer Tools provides powerful debugging capabilities that can help identify and fix issues in React components. Here are some techniques for debugging React components using React Developer Tools:
1. Setting breakpoints: You can set breakpoints in your code directly from the React Component Tree view. Simply right-click on a component and select “Break on > All Updates” or “Break on > Render”. This will pause the execution of your code whenever that component is updated or rendered, allowing you to inspect the component’s props and state at that point in time.
2. Inspecting component updates: React Developer Tools allows you to track component updates and see what triggered them. In the “Components” tab, you can enable the “Highlight Updates” option, which will highlight components that have been updated since the last render. This can help identify unnecessary re-renders and optimize performance.
3. Console logging: You can log messages to the browser console directly from React Developer Tools. Simply select a component in the React Component Tree view, right-click, and choose “Log Component”. This will log the selected component to the console, along with its props and state. You can also log specific values or expressions by selecting them in the “Props” or “State” tabs and choosing “Log Value”.
Using React Developer Tools to Optimize Performance
React Developer Tools provides several features that can help optimize the performance of your React application. Here are some techniques for using React Developer Tools to optimize performance:
1. Profiling: React Developer Tools includes a built-in profiler that allows you to measure the performance of your components. To start profiling, click on the “Profiler” tab in the developer tools panel. Then, interact with your application to trigger different actions or events. The profiler will record the time taken by each component to render and update. This information can be used to identify performance bottlenecks and optimize slow components.
2. Highlighting unnecessary re-renders: As mentioned earlier, React Developer Tools can highlight components that have been updated since the last render. This can help identify unnecessary re-renders caused by inefficient code or unnecessary prop changes. By optimizing these re-renders, you can improve the overall performance of your application.
3. Analyzing component dependencies: React Developer Tools allows you to analyze the dependencies between components in your application. By selecting a component in the React Component Tree view and clicking on the “Dependencies” tab, you can see which components are consuming its props. This can help identify components that are unnecessarily re-rendering due to changes in their dependencies. By optimizing these dependencies, you can reduce the number of re-renders and improve performance.
Debugging React Native Applications with React Developer Tools
React Developer Tools can also be used to debug React Native applications. The process is similar to debugging web applications, but with a few additional steps.
To debug a React Native application using React Developer Tools, follow these steps:
1. Enable remote debugging in your React Native application by adding the following line of code to your entry file (usually `index.js` or `App.js`):
“`javascript
import { AppRegistry } from ‘react-native’;
import App from ‘./App’;
AppRegistry.registerComponent(‘YourAppName’, () => App);
AppRegistry.runApplication(‘YourAppName’, {
rootTag: document.getElementById(‘root’),
});
“`
2. Open your React Native application in a simulator or on a physical device.
3. Open the developer tools panel in your browser and navigate to the “React” tab.
4. Click on the “Select a view” button and choose your React Native application from the list.
5. You should now see the React Component Tree and other debugging features specific to React Native.
Advanced Features of React Developer Tools
In addition to the basic features we have discussed so far, React Developer Tools also offers some advanced features that can further enhance your development workflow. Here are a few examples:
1. Time-travel debugging: React Developer Tools allows you to time-travel through the state and props changes of your components. This can be useful for understanding how the component’s state evolves over time and for reproducing specific scenarios or bugs.
2. Editing component props and state: With React Developer Tools, you can edit the props and state of a component directly from the developer tools panel. This can be helpful for testing different scenarios or making quick changes to the component’s behavior.
3. Exporting and importing component snapshots: React Developer Tools allows you to export and import snapshots of your components. This can be useful for sharing component states or for saving and restoring specific application states during development.
Tips and Tricks for Using React Developer Tools
Here are some tips and tricks for using React Developer Tools more efficiently:
1. Use keyboard shortcuts: React Developer Tools provides several keyboard shortcuts that can speed up your workflow. For example, you can press “Ctrl + P” (or “Cmd + P” on Mac) to quickly search for a specific component in the React Component Tree view.
2. Customize the display: You can customize the display of React Developer Tools to suit your preferences. For example, you can change the theme, adjust the font size, or hide certain panels that you don’t use frequently.
3. Use the “Inspect” mode: React Developer Tools includes an “Inspect” mode that allows you to select a component directly from your application’s U
Simply right-click on an element in your application and choose “Inspect” to select the corresponding component in the React Component Tree view.
Conclusion and Next Steps for Improving Your React Development Workflow
In conclusion, React Developer Tools is a powerful tool that provides a wide range of features for inspecting, debugging, and optimizing React components. By using React Developer Tools, you can gain a deeper understanding of your application’s component hierarchy, inspect component properties and state, debug issues more effectively, optimize performance, and even debug React Native applications.
To further improve your React development workflow, consider exploring more advanced features of React Developer Tools, experimenting with different debugging techniques, and staying up-to-date with the latest updates and improvements in the tool. By leveraging the full potential of React Developer Tools, you can become a more efficient and effective React developer.
Mastering React Development: Enhance Your Skills with Top-notch Training
React is a popular JavaScript library for building user interfaces. It was developed by Facebook and has gained immense popularity in the web development community. React allows developers to build reusable UI components that can be easily composed to create complex user interfaces. One of the key features of React is its virtual DOM, which allows for efficient rendering and updating of components.
React’s popularity can be attributed to its simplicity and flexibility. It provides a declarative syntax for defining UI components, making it easier to understand and maintain code. React also encourages the use of reusable components, which can save developers time and effort when building applications.
In addition to its core library, React has a vast ecosystem of tools and libraries that enhance its functionality. These include state management libraries like Redux and MobX, routing libraries like React Router, and testing frameworks like Jest. These tools make it easier for developers to build complex applications with React.
Key Takeaways
- React is a popular JavaScript library for building user interfaces.
- React components are reusable building blocks that can be composed to create complex UIs.
- React state management allows for dynamic updates to the UI based on user interactions or data changes.
- React routing and navigation enable users to move between different pages or views within a single-page application.
- React hooks and context API provide a way to manage state and share data between components without using class components.
Understanding React Components
In React, components are the building blocks of user interfaces. They are reusable pieces of code that encapsulate the logic and presentation of a part of the U
Components can be either functional or class-based.
Functional components are simple JavaScript functions that return JSX (JavaScript XML), which is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. Functional components are easier to read and test, and they are recommended for most use cases.
Class components are ES6 classes that extend the React.Component class. They have additional features like lifecycle methods and access to state and props. Class components are useful when you need to manage state or use lifecycle methods.
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. It is used to define the structure and appearance of React components. JSX makes it easier to write and understand code by combining HTML-like syntax with JavaScript logic.
React State Management
State Management Library | Popularity | Learning Curve | Performance |
---|---|---|---|
Redux | Very popular | Steep | High performance |
MobX | Popular | Easy | High performance |
Context API | Increasingly popular | Easy | Medium performance |
State is an important concept in React. It represents the data that a component needs to render and update itself. State is mutable and can be changed using the setState method.
The setState method is used to update the state of a component. It takes an object as an argument, which contains the new values for the state properties. When setState is called, React re-renders the component with the updated state.
In addition to the built-in state management in React, there are also state management libraries like Redux and Mob
These libraries provide a centralized way to manage state in larger applications. They allow you to define actions and reducers to update and retrieve state, making it easier to manage complex application state.
React Routing and Navigation
Routing and navigation are important aspects of building web applications. In React, routing is handled by a library called React Router. React Router provides a declarative way to define routes and navigate between them.
React Router allows you to define routes using the Route component. Each route is associated with a specific component that will be rendered when the route matches the current URL. React Router also provides features like nested routes, dynamic routing, and route parameters.
Nested routes allow you to define routes within other routes. This is useful when you have components that need to be rendered within a parent component. Dynamic routing allows you to define routes with dynamic segments, which can be used to pass data or parameters to the rendered component.
React Hooks and Context API
React Hooks are a new feature introduced in React 16.8 that allows you to use state and other React features without writing class components. Hooks provide a simpler and more intuitive way to manage state and side effects in functional components.
The useState hook allows you to add state to functional components. It takes an initial value as an argument and returns an array with two elements: the current state value and a function to update the state.
The useEffect hook allows you to perform side effects in functional components. Side effects include things like fetching data, subscribing to events, or manually changing the DOM. The useEffect hook takes a function as an argument and runs it after every render.
The useContext hook allows you to access the value of a context directly in a functional component. The Context API is a feature of React that allows you to share data between components without passing props manually.
Advanced React Concepts
In addition to the basic concepts of React, there are also more advanced concepts that can be used to build more complex applications.
Server-side rendering (SSR) is a technique that allows you to render React components on the server and send the HTML to the client. This can improve performance and SEO, as the initial page load is faster and search engines can index the content.
Code splitting is a technique that allows you to split your code into smaller chunks and load them on demand. This can improve performance by reducing the initial load time of your application.
Lazy loading is a technique that allows you to load components or resources only when they are needed. This can improve performance by reducing the amount of code and resources that need to be loaded initially.
HOCs (Higher-Order Components) are functions that take a component as an argument and return a new component with additional functionality. HOCs are useful for adding common functionality to multiple components.
Render props is a pattern where a component accepts a function as a prop and calls it with its internal state or other data. This allows for greater flexibility and reusability of components.
React Native is a framework for building mobile applications using React. It allows you to write code once and deploy it on multiple platforms, including iOS and Android.
Building Real-world React Applications
Building real-world applications with React involves understanding common application architectures and patterns.
One common architecture is the component-based architecture, where the UI is divided into reusable components. This allows for easier maintenance and reusability of code.
Another common pattern is the container/component pattern, where container components are responsible for fetching data and managing state, while presentational components are responsible for rendering the U
There are also popular React frameworks like Next.js and Gatsby that provide additional features and functionality for building real-world applications. Next.js is a framework for server-rendered React applications, while Gatsby is a framework for building static websites.
Testing and Debugging React Applications
Testing and debugging are important aspects of building reliable and bug-free applications.
Jest is a popular testing framework for React applications. It provides a simple and intuitive API for writing tests and comes with built-in support for mocking and code coverage.
Enzyme is a testing utility for React that provides a set of APIs for interacting with React components. It allows you to simulate events, query the rendered output, and assert on the component’s state and props.
React Developer Tools is a browser extension that allows you to inspect and debug React components in the browser. It provides a tree view of the component hierarchy, as well as tools for inspecting props, state, and performance.
Chrome DevTools is a set of web developer tools built into the Chrome browser. It provides tools for debugging JavaScript, inspecting network requests, profiling performance, and more.
Optimizing React Performance
Performance optimization is important to ensure that your React application runs smoothly and efficiently.
React Profiler is a tool that allows you to measure the performance of your React components. It provides insights into how long each component takes to render, as well as how often they are rendered.
There are also performance monitoring tools like New Relic and Datadog that can help you identify performance bottlenecks in your application. These tools provide real-time monitoring of your application’s performance metrics, allowing you to identify and fix issues quickly.
Deploying React Applications to Production
Deploying a React application to production involves hosting the application on a server and making it accessible to users.
There are popular hosting platforms like Netlify and Heroku that provide easy deployment options for React applications. These platforms allow you to deploy your application with a single command and provide features like automatic SSL, CDN, and continuous deployment.
CI/CD (Continuous Integration/Continuous Deployment) pipelines are a set of practices and tools that automate the process of building, testing, and deploying software. They allow you to automate the deployment of your React application, ensuring that it is always up-to-date and bug-free.
Mastering React: Essential Skills for a Top-Notch Developer
React is a JavaScript library that was developed by Facebook in 2013. It quickly gained popularity among web developers due to its simplicity and efficiency in building user interfaces. React allows developers to create reusable UI components, making it easier to manage and update complex web applications.
One of the main advantages of using React in web development is its virtual DOM (Document Object Model) implementation. The virtual DOM is a lightweight copy of the actual DOM, which allows React to efficiently update only the necessary parts of the UI when changes occur. This results in faster rendering and improved performance compared to traditional JavaScript frameworks.
Many popular companies are using React in their web development projects. Facebook, Instagram, Netflix, Airbnb, and WhatsApp are just a few examples. These companies have chosen React for its ability to handle large amounts of data and provide a seamless user experience.
Key Takeaways
- React is an important tool in web development
- Components, props, and state are core concepts in React
- React allows for building interactive UIs with event handling, forms, and validation
- React Router enables navigation and routing in single-page applications
- Redux manages state and data flow in React applications
Understanding the Core Concepts of React: Components, Props, and State
Components are the building blocks of a React application. They are reusable pieces of code that encapsulate the UI logic and can be composed together to create complex user interfaces. Components can be either functional or class-based.
Props (short for properties) are used to pass data from a parent component to its child components. They are read-only and cannot be modified by the child components. Props allow for easy communication between components and enable the creation of dynamic and interactive UIs.
State is used to manage the internal data of a component. Unlike props, state can be modified by the component itself. When the state of a component changes, React automatically re-renders the component and updates the UI accordingly.
To illustrate how components, props, and state work together in React, let’s consider an example of a simple counter component. The counter component has a state variable called “count” that keeps track of the current count value. The count value is initially set to 0 and can be incremented or decremented by clicking on buttons.
“`javascript
import React, { useState } from ‘react’;
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count – 1);
};
return (
Counter: {count}
);
};
export default Counter;
“`
In this example, the count value is stored in the state using the useState hook. The increment and decrement functions modify the count value by calling the setCount function. The current count value is displayed in the UI using JSX syntax.
Building Interactive UIs with React: Event Handling, Forms, and Validation
Topic | Description |
---|---|
Event Handling | Learn how to handle events in React, such as onClick, onChange, onSubmit, etc. |
Forms | Understand how to create and manage forms in React, including controlled and uncontrolled components. |
Validation | Explore different ways to validate user input in React, such as using regular expressions, third-party libraries, or custom validation functions. |
Event handling in React is similar to event handling in traditional JavaScript. However, React uses synthetic events instead of native browser events to ensure consistent behavior across different browsers.
To handle events in React, you can use the onClick, onChange, or onSubmit attributes on HTML elements. These attributes take a callback function that will be executed when the event occurs.
Creating forms in React is straightforward. You can use the HTML form elements such as input, textarea, and select, and handle their values using state. When a user interacts with a form element, you can update the corresponding state variable to reflect the changes.
Validating user input in React can be done by adding conditional logic to check if the input meets certain criteria. You can display error messages or disable form submission until all input fields are valid.
“`javascript
import React, { useState } from ‘react’;
const LoginForm = () => {
const [username, setUsername] = useState(”);
const [password, setPassword] = useState(”);
const [error, setError] = useState(”);
const handleUsernameChange = (event) => {
setUsername(event.target.value);
};
const handlePasswordChange = (event) => {
setPassword(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
if (username === ‘admin’ && password === ‘password’) {
setError(”);
// Perform login logic
} else {
setError(‘Invalid username or password’);
}
};
return (
);
};
export default LoginForm;
“`
In this example, the LoginForm component handles the username and password input fields using state variables. The handleUsernameChange and handlePasswordChange functions update the corresponding state variables when the input values change. The handleSubmit function is called when the form is submitted, and it performs the login logic by checking if the username and password are valid.
Working with React Router: Navigation and Routing in Single-Page Applications
React Router is a popular library for handling navigation and routing in single-page applications built with React. It allows you to define routes and render different components based on the current URL.
To use React Router, you need to install it as a dependency in your project. You can then define routes using the Route component and specify the component to render for each route.
“`javascript
import React from ‘react’;
import { BrowserRouter as Router, Route, Link } from ‘react-router-dom’;
const Home = () =>
Home
;
const About = () =>
About
;
const Contact = () =>
Contact
;
const App = () => (
);
export default App;
“`
In this example, the App component defines three routes: one for the home page, one for the about page, and one for the contact page. The Link component is used to create navigation links that will update the URL and render the corresponding component.
Managing Data with Redux: State Management and Data Flow in React Applications
Redux is a state management library that can be used with React to manage the state of an application. It provides a predictable and centralized way to manage data, making it easier to debug and test complex applications.
In Redux, the state of an application is stored in a single JavaScript object called the store. The store is immutable, meaning that it cannot be directly modified. Instead, you need to dispatch actions to update the state.
Actions are plain JavaScript objects that describe what happened in the application. They are dispatched using the store’s dispatch function and are handled by reducers.
Reducers are pure functions that take the current state and an action as arguments and return a new state. They specify how the state should be updated based on the action that was dispatched.
“`javascript
import { createStore } from ‘redux’;
// Define the initial state
const initialState = {
count: 0,
};
// Define the reducer function
const reducer = (state = initialState, action) => {
switch (action.type) {
case ‘INCREMENT’:
return { …state, count: state.count + 1 };
case ‘DECREMENT’:
return { …state, count: state.count – 1 };
default:
return state;
}
};
// Create the store
const store = createStore(reducer);
// Dispatch actions to update the state
store.dispatch({ type: ‘INCREMENT’ });
store.dispatch({ type: ‘INCREMENT’ });
store.dispatch({ type: ‘DECREMENT’ });
// Get the current state
const currentState = store.getState();
console.log(currentState); // { count: 1 }
“`
In this example, we define an initial state object with a count property set to 0. We then define a reducer function that handles two types of actions: INCREMENT and DECREMENT. When an INCREMENT action is dispatched, the count property is incremented by 1. When a DECREMENT action is dispatched, the count property is decremented by 1.
We create the store using the createStore function from Redux. We can then dispatch actions using the store’s dispatch function and get the current state using the store’s getState function.
Building Dynamic and Responsive UIs with React Hooks
React Hooks were introduced in React 16.8 as a way to use state and other React features without writing class components. Hooks allow you to reuse stateful logic between components and make it easier to manage complex UIs.
There are several built-in hooks in React, such as useState, useEffect, useContext, and useRef. The useState hook allows you to add state to functional components, while the useEffect hook allows you to perform side effects, such as fetching data or subscribing to events.
“`javascript
import React, { useState, useEffect } from ‘react’;
const Timer = () => {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds((prevSeconds) => prevSeconds + 1);
}, 1000);
return () => {
clearInterval(interval);
};
}, []);
return
Seconds: {seconds}
;
};
export default Timer;
“`
In this example, the Timer component uses the useState hook to add a seconds state variable. The useEffect hook is used to start a timer that increments the seconds value every second. The useEffect hook also returns a cleanup function that clears the interval when the component is unmounted.
Testing React Applications: Unit Testing and Integration Testing Techniques
Testing is an important part of building robust and reliable React applications. There are two main types of testing in React: unit testing and integration testing.
Unit testing involves testing individual components or functions in isolation to ensure that they work correctly. You can use testing libraries such as Jest and React Testing Library to write unit tests for your React components.
Integration testing involves testing how different components or modules work together as a whole. It ensures that the different parts of your application integrate correctly and produce the expected results. You can use tools like Cypress or Selenium for integration testing in React applications.
Here’s an example of a unit test for a simple Counter component using Jest and React Testing Library:
“`javascript
import React from ‘react’;
import { render, fireEvent } from ‘@testing-library/react’;
import Counter from ‘./Counter’;
test(‘increments the count when the increment button is clicked’, () => {
const { getByText } = render(
const incrementButton = getByText(‘Increment’);
const countElement = getByText(‘Counter: 0’);
fireEvent.click(incrementButton);
expect(countElement.textContent).toBe(‘Counter: 1’);
});
“`
In this example, we render the Counter component using the render function from React Testing Library. We then use the getByText function to get references to the increment button and the count element. We simulate a click event on the increment button using the fireEvent.click function. Finally, we assert that the count element’s text content is updated correctly.
Optimizing Performance in React Applications: Best Practices and Tools
Optimizing performance is crucial for delivering a fast and responsive user experience in React applications. There are several best practices and tools that can help you optimize the performance of your React applications.
Some best practices for optimizing performance in React applications include:
– Minimizing unnecessary re-renders by using shouldComponentUpdate or React.memo.
– Using lazy loading and code splitting to load components and resources only when needed.
– Avoiding unnecessary calculations or expensive operations in render methods.
– Using memoization techniques to cache expensive function calls or calculations.
There are also several tools available for measuring and improving performance in React applications. Some popular tools include:
– React DevTools: A browser extension that allows you to inspect and debug React components, including their props, state, and rendered output.
– Lighthouse: A tool built into Google Chrome that audits web pages for performance, accessibility, and other best practices.
– Webpack Bundle Analyzer: A tool that analyzes your Webpack bundle and provides insights into its size and composition.
– Performance Timeline: A feature of the Chrome DevTools that allows you to record and analyze the performance of your web application.
Here’s an example of using React.memo to optimize the rendering of a component:
“`javascript
import React from ‘react’;
const ExpensiveComponent = ({ data }) => {
// Expensive calculations or operations here
return
;
};
export default React.memo(ExpensiveComponent);
“`
In this example, the ExpensiveComponent performs some expensive calculations or operations. By wrapping the component with React.memo, React will memoize the component and only re-render it if the props have changed. This can help improve performance by avoiding unnecessary re-renders.
Deploying React Applications: Strategies and Tools for Production Environments
Deploying a React application involves making it available to users on the internet. There are several strategies and tools that can help you deploy your React applications in production environments.
Some common strategies for deploying React applications include:
– Hosting on a static file server: You can build your React application using tools like Webpack or Create React App and deploy the resulting static files to a file server, such as Amazon S3 or Netlify.
– Deploying to a cloud platform: Cloud platforms like AWS, Google Cloud, and Microsoft Azure provide services for hosting and deploying web applications. You can use services like AWS Elastic Beanstalk, Google App Engine, or Azure App Service to deploy your React application.
– Using a content delivery network (CDN): CDNs like Cloudflare or Fastly can cache your static assets and distribute them across multiple servers worldwide, improving the performance and availability of your React application.
There are also several tools available that can help you automate the deployment process and streamline your workflow. Some popular tools include Jenkins, Ansible, Puppet, Chef, and Docker. Jenkins is a widely used open-source automation server that allows you to automate the building, testing, and deployment of your applications. Ansible is a powerful automation tool that allows you to define and manage your infrastructure as code. Puppet and Chef are configuration management tools that help you automate the deployment and management of your infrastructure. Docker is a containerization platform that allows you to package your applications and their dependencies into lightweight, portable containers, making it easier to deploy and scale your applications. These tools can greatly simplify the deployment process and improve the efficiency of your workflow.
Boost Your Web Development Project with a Skilled React Developer: How to Hire the Best
React is a JavaScript library that is widely used in web development projects. It was developed by Facebook and is known for its efficiency and flexibility in building user interfaces. React allows developers to create reusable UI components, making it easier to maintain and update web applications.
One of the main benefits of using React in web development projects is its virtual DOM (Document Object Model) feature. The virtual DOM allows React to efficiently update only the necessary parts of a web page, rather than reloading the entire page. This results in faster rendering and improved performance, especially for complex and dynamic web applications.
Another advantage of using React is its component-based architecture. With React, developers can break down the user interface into smaller, reusable components. This makes it easier to manage and maintain the codebase, as well as promote code reusability. Additionally, React’s component-based architecture allows for better collaboration among developers, as they can work on different components simultaneously without interfering with each other’s code.
Key Takeaways
- React development is important in web development projects due to its ability to create dynamic and interactive user interfaces.
- Hiring a skilled React developer can benefit your web development project by improving its efficiency, scalability, and user experience.
- Assessing your web development needs and determining the necessary skills can help you find the right React developer for your project.
- Skilled React developers can be found through online job boards, social media, and referrals from colleagues and industry contacts.
- Evaluating a React developer’s technical skills and experience, as well as their soft skills, is crucial in ensuring a successful hire for your web development project.
Benefits of Hiring a Skilled React Developer for Your Web Development Project
Hiring a skilled React developer for your web development project is crucial for its success. A skilled React developer has a deep understanding of the library and can leverage its features to build efficient and high-performing web applications.
One of the advantages of having a skilled React developer on your team is their ability to write clean and maintainable code. React encourages best practices such as modularization and reusability, and a skilled developer will be able to follow these practices effectively. Clean and maintainable code is easier to understand, debug, and update, which ultimately leads to a more efficient development process.
Another benefit of hiring a skilled React developer is their ability to optimize performance. React provides various tools and techniques for optimizing performance, such as lazy loading, code splitting, and memoization. A skilled developer will be able to identify performance bottlenecks and implement the necessary optimizations to ensure a smooth and fast user experience.
Furthermore, a skilled React developer can contribute to the overall architecture and design of the web application. They can make informed decisions on how to structure the codebase, manage state, and handle data flow. This level of expertise is crucial for building scalable and maintainable web applications that can easily accommodate future updates and enhancements.
How to Assess Your Web Development Needs and Determine the Skills Required
Before hiring a React developer, it is important to assess your web development needs and determine the specific skills required for your project. This will help you find a developer who is the right fit for your requirements and can contribute effectively to the project’s success.
Start by identifying the goals and objectives of your web development project. What are you trying to achieve with the application? What features and functionalities do you need? Understanding your project’s requirements will give you a clear idea of the skills and expertise needed from a React developer.
Next, consider the complexity of your web application. Is it a simple website or a complex web application? Does it require integration with external APIs or databases? The complexity of your project will determine the level of expertise required from a React developer. For more complex projects, you may need someone with experience in handling data flow, state management, and performance optimization.
Additionally, consider the timeline and budget of your project. If you have strict deadlines or a limited budget, you may need a React developer who can work efficiently under pressure and deliver high-quality code within the given constraints.
Where to Find Skilled React Developers for Your Web Development Project
Source | Pros | Cons |
---|---|---|
Large pool of professionals, easy to filter by skills and experience | May require premium account to message candidates, high competition from other recruiters | |
GitHub | Access to developers’ code and projects, can assess technical skills | Not all developers have a GitHub profile, may not be actively looking for work |
Job boards (Indeed, Glassdoor, etc.) | Wide reach, candidates actively looking for work | May receive a high volume of unqualified applicants, may require payment to post job listings |
Referrals | Pre-screened candidates, potential for cultural fit | May not have a large pool of candidates, potential for bias in referrals |
Freelance platforms (Upwork, Freelancer, etc.) | Access to global talent, can hire on a project basis | May require more management and communication, quality of work may vary |
There are several sources where you can find skilled React developers for your web development project. Each source has its own pros and cons, so it’s important to consider them before making a decision.
One of the most common sources for finding skilled React developers is online job platforms such as Upwork, Freelancer, and Toptal. These platforms allow you to post job listings and connect with developers from around the world. The advantage of using online job platforms is that you have access to a large pool of talent and can choose from a wide range of skills and experience levels. However, the downside is that it can be time-consuming to sift through the applications and conduct interviews.
Another source for finding skilled React developers is through referrals from your professional network. Reach out to colleagues, friends, or industry contacts who have worked with React developers in the past and ask for recommendations. Referrals are often reliable because they come from trusted sources who have firsthand experience working with the developers. However, the downside is that the pool of candidates may be limited compared to online job platforms.
You can also consider hiring a React development agency or outsourcing the project to a software development company. These companies have a team of skilled React developers who can work on your project. The advantage of working with an agency or company is that they have a structured hiring process and can provide ongoing support and maintenance for your web application. However, the downside is that it may be more expensive compared to hiring an individual developer.
How to Evaluate a React Developer’s Technical Skills and Experience
When evaluating a React developer’s technical skills and experience, there are several key factors to consider. These factors will help you determine if the developer has the necessary expertise to handle your web development project.
Firstly, consider the developer’s proficiency in React. Ask about their experience with React and how long they have been working with the library. A skilled React developer should have a deep understanding of React’s core concepts, such as components, state management, and lifecycle methods.
Next, assess their knowledge of JavaScript. React is built on top of JavaScript, so it’s important for a React developer to have a strong foundation in JavaScript programming. Ask about their experience with JavaScript frameworks and libraries, such as Angular or Vue.js, as this can indicate their overall proficiency in JavaScript.
Additionally, consider their experience with related technologies and tools. React is often used in conjunction with other technologies, such as Redux for state management or GraphQL for data fetching. A skilled React developer should have experience working with these technologies and be able to integrate them seamlessly into the web application.
Furthermore, evaluate their understanding of web development best practices and standards. Ask about their knowledge of HTML, CSS, and responsive design principles. A skilled React developer should be able to write clean and semantic HTML code, style components using CSS or CSS-in-JS, and ensure that the web application is accessible and responsive across different devices.
Essential Soft Skills to Look for in a React Developer for Your Web Development Project
In addition to technical skills, it is important to consider the soft skills of a React developer when hiring for your web development project. Soft skills are personal attributes that enable effective communication, collaboration, and problem-solving.
One of the essential soft skills to look for in a React developer is strong communication skills. A skilled React developer should be able to effectively communicate their ideas, ask clarifying questions, and provide updates on the progress of the project. Good communication skills are crucial for collaborating with other team members, understanding client requirements, and resolving any issues that may arise during the development process.
Another important soft skill is problem-solving ability. A skilled React developer should have a logical and analytical mindset that allows them to identify and solve problems efficiently. They should be able to think critically, troubleshoot issues, and come up with creative solutions to technical challenges. Problem-solving ability is especially important in web development projects where unexpected issues can arise at any stage of the development process.
Furthermore, consider their ability to work well in a team. Web development projects often involve collaboration with designers, backend developers, project managers, and other stakeholders. A skilled React developer should be able to work effectively in a team environment, contribute to discussions, and provide constructive feedback. They should also be open to learning from others and sharing their knowledge and expertise with the team.
How to Conduct a Successful Interview with a React Developer for Your Web Development Project
Conducting a successful interview with a React developer is crucial for assessing their skills, experience, and fit for your web development project. Here are some tips to help you conduct an effective interview:
1. Prepare a list of interview questions: Before the interview, prepare a list of questions that will help you assess the developer’s technical skills, experience, and problem-solving ability. Ask about their experience with React, their approach to handling complex projects, and how they have solved technical challenges in the past.
2. Include coding exercises or challenges: Consider including coding exercises or challenges as part of the interview process. This will allow you to assess the developer’s coding skills, problem-solving ability, and familiarity with React. Provide them with a small coding task or ask them to solve a specific problem using React.
3. Ask for examples of past projects: Request the developer to provide examples of past projects they have worked on using React. This will give you an idea of their coding style, attention to detail, and ability to deliver high-quality work. Ask them to explain their role in the project and any challenges they faced during the development process.
4. Assess their ability to explain technical concepts: During the interview, ask the developer to explain technical concepts related to React and web development. This will help you gauge their understanding of the library and their ability to communicate complex ideas in a clear and concise manner.
5. Consider cultural fit: In addition to technical skills, consider the cultural fit of the developer within your team. Assess their attitude, work ethic, and willingness to learn and adapt. A good cultural fit is important for fostering a positive work environment and ensuring effective collaboration among team members.
Negotiating the Salary and Terms of Employment for Your React Developer
Negotiating the salary and terms of employment for your React developer is an important step in the hiring process. Here are some factors to consider when negotiating:
1. Market rates: Research the market rates for React developers in your area or industry. This will give you an idea of the average salary range and help you determine a fair offer. Consider factors such as experience, skills, and demand for React developers when setting the salary.
2. Benefits and perks: In addition to salary, consider the benefits and perks you can offer to attract and retain top talent. This can include health insurance, retirement plans, flexible working hours, remote work options, or professional development opportunities. Highlight these benefits during the negotiation process to make your offer more attractive.
3. Scope of work: Discuss the scope of work and responsibilities of the React developer. Clarify their role in the project, the tasks they will be responsible for, and any specific deliverables or milestones they need to meet. This will help set clear expectations and ensure that both parties are on the same page.
4. Contract terms: Discuss the terms of employment, such as the duration of the contract, probation period, notice period, and any non-compete or confidentiality agreements. Ensure that both parties are comfortable with the terms and that they are legally binding.
5. Flexibility: Be open to negotiation and consider any requests or concerns raised by the React developer. Flexibility in negotiating terms can help build a positive relationship and increase the chances of securing top talent for your web development project.
Onboarding Your React Developer and Ensuring a Smooth Integration into Your Web Development Team
Onboarding a new React developer is crucial for ensuring a smooth integration into your web development team. Here are some best practices for onboarding:
1. Provide a clear onboarding plan: Create a detailed onboarding plan that outlines the tasks, responsibilities, and expectations for the new React developer. This will help them understand their role in the project and what is expected of them.
2. Assign a mentor or buddy: Assign a mentor or buddy to the new React developer. This person can provide guidance, answer questions, and help them navigate the team dynamics. The mentor should be someone experienced in React development who can provide technical support and share best practices.
3. Conduct knowledge transfer sessions: Organize knowledge transfer sessions where the new React developer can learn about the existing codebase, project architecture, and development processes. This will help them get up to speed quickly and understand how their work fits into the larger project.
4. Encourage collaboration and communication: Foster a culture of collaboration and open communication within your web development team. Encourage the new React developer to ask questions, share ideas, and actively participate in discussions. This will help them feel valued and integrated into the team.
5. Provide ongoing support and feedback: Offer ongoing support and feedback to the new React developer. Schedule regular check-ins to discuss their progress, address any concerns or challenges they may have, and provide constructive feedback on their work. This will help them improve their skills and contribute effectively to the project.
Best Practices for Managing and Retaining Your Skilled React Developer for Long-Term Success
Managing and retaining a skilled React developer is crucial for long-term success in your web development project. Here are some best practices to consider:
1. Provide growth opportunities: Offer opportunities for professional growth and development to your React developer. This can include attending conferences or workshops, participating in online courses or certifications, or working on challenging projects that allow them to expand their skills and knowledge.
2. Recognize and reward achievements: Recognize and reward your React developer’s achievements and contributions to the project. This can be done through verbal praise, bonuses, promotions, or other forms of recognition. Acknowledging their hard work and dedication will motivate them to continue performing at a high level.
3. Foster a positive work environment: Create a positive work environment that promotes collaboration, creativity, and work-life balance. Encourage open communication, provide opportunities for team bonding, and ensure that the workload is manageable and realistic. A positive work environment will help retain your skilled React developer and attract other top talent to your team.
4. Offer competitive compensation: Regularly review and adjust the salary and benefits of your React developer to ensure that they are competitive in the market. Stay updated on industry trends and market rates to ensure that you are offering a fair and attractive compensation package.
5. Provide ongoing feedback and support: Continue to provide ongoing feedback and support to your React developer. Schedule regular performance reviews to discuss their progress, provide constructive feedback, and set goals for the future. Offer support and resources to help them overcome any challenges they may face in their role.
In conclusion, React development plays a crucial role in web development projects due to its efficiency, flexibility, and component-based architecture. Hiring a skilled React developer is essential for the success of your web development project as they can contribute their expertise in writing clean code, optimizing performance, and making informed architectural decisions. When assessing your web development needs, it is important to determine the specific skills required based on the project’s goals, complexity, timeline, and budget. Skilled React developers can be found through online job platforms, freelance websites, or by working with a reputable software development agency. It is important to thoroughly evaluate candidates by reviewing their portfolios, assessing their technical skills through coding tests or interviews, and checking their references. Additionally, communication and collaboration skills are also important factors to consider when hiring a React developer, as they will need to work closely with other team members and stakeholders throughout the development process. By investing in a skilled React developer, you can ensure that your web development project is executed efficiently and effectively, resulting in a high-quality and user-friendly website or application.
Unveiling the Role of a React Developer: A Comprehensive Job Description
React is a popular JavaScript library for building user interfaces. It was developed by Facebook and has gained immense popularity in the web development community. React allows developers to build reusable UI components, making it easier to create complex web applications. Its popularity can be attributed to its simplicity, performance, and flexibility.
In today’s digital age, where user experience is paramount, React plays a crucial role in building modern web applications. With React, developers can create interactive and dynamic user interfaces that are responsive and user-friendly. It allows for efficient rendering of components, resulting in faster load times and improved performance. React also provides a virtual DOM (Document Object Model), which allows for efficient updates to the UI without the need for a full page reload.
Key Takeaways
- React development is a popular and in-demand skill in the web development industry.
- Essential skills for a React developer include proficiency in JavaScript, HTML, and CSS, as well as knowledge of React libraries and frameworks.
- The role of a React developer involves designing and implementing user interfaces, collaborating with designers and backend developers, and troubleshooting applications.
- Key tasks and deliverables of a React developer include creating reusable components, optimizing application performance, and ensuring cross-browser compatibility.
- Collaboration with designers and backend developers is crucial for building responsive and user-friendly web applications.
Essential Skills and Qualifications for a React Developer
To become a successful React developer, there are certain technical skills and qualifications that are essential. Firstly, a strong foundation in JavaScript is crucial as React is built on top of it. Understanding concepts such as variables, functions, loops, and arrays is essential for writing clean and efficient code.
In addition to JavaScript, knowledge of HTML and CSS is also important as these are the building blocks of web development. Familiarity with React libraries and frameworks such as Redux, React Router, and Next.js is also beneficial as they provide additional functionality and simplify the development process.
Apart from technical skills, soft skills are equally important for a React developer. Good communication skills are essential for collaborating with other team members and understanding project requirements. Problem-solving skills are also crucial as developers often encounter challenges while building complex applications. Lastly, teamwork is important as React developers often work in cross-functional teams where collaboration and cooperation are key.
Understanding the Role and Responsibilities of a React Developer
The role of a React developer in a web development team is to build user interfaces using the React library. They work closely with designers to implement the visual elements of a website or application and collaborate with backend developers to integrate the frontend with the backend APIs and databases.
The responsibilities of a React developer include writing clean and efficient code, building reusable components and UI elements, and ensuring the responsiveness and user-friendliness of the application. They are also responsible for testing and debugging the application to ensure its functionality and performance.
Collaboration with other team members is crucial for a React developer. They need to communicate effectively with designers to understand the design requirements and implement them in React. They also need to work closely with backend developers to ensure seamless integration between the frontend and backend of the application.
Key Tasks and Deliverables of a React Developer
Task/Deliverable | Description |
---|---|
Developing Components | Creating reusable and modular components using React library. |
Implementing State Management | Using state management libraries like Redux or Context API to manage application state. |
Integrating APIs | Fetching data from APIs and integrating it into the application using libraries like Axios or Fetch. |
Testing Components | Writing unit tests for components using testing libraries like Jest and Enzyme. |
Optimizing Performance | Identifying and resolving performance issues in the application using tools like React Profiler. |
Deploying Application | Deploying the application to production environment using tools like Heroku or Netlify. |
The key tasks of a React developer include building reusable components and UI elements, integrating with backend APIs and databases, and testing and debugging React applications.
Building reusable components is one of the core tasks of a React developer. By creating reusable components, developers can save time and effort by reusing code across different parts of the application. This not only improves efficiency but also ensures consistency in the user interface.
Integrating with backend APIs and databases is another important task for a React developer. They need to communicate with backend developers to understand the API endpoints and data structures. By integrating the frontend with the backend, they can ensure that data is fetched and displayed correctly in the user interface.
Testing and debugging are crucial steps in the development process. A React developer needs to test their code to ensure its functionality and performance. They also need to debug any issues that arise during testing or in production to ensure a smooth user experience.
Collaborating with Designers and Backend Developers
Collaboration with designers is essential for a React developer as they need to implement the visual elements of a website or application. They need to understand the design requirements provided by designers and translate them into code using React. Effective communication is key in this collaboration to ensure that the design is implemented accurately.
Collaboration with backend developers is also important for a React developer. They need to work closely with backend developers to integrate the frontend with the backend APIs and databases. This collaboration ensures that data is fetched and displayed correctly in the user interface and that the application functions as intended.
Effective collaboration with both designers and backend developers is crucial for a React developer to ensure a seamless and cohesive development process. By working together, they can create a user-friendly and functional web application.
Building Responsive and User-Friendly Web Applications
Building responsive and user-friendly web applications is essential in today’s digital landscape. Users expect websites and applications to be accessible on different devices and screen sizes. React provides several techniques for building responsive web applications.
One technique is using media queries in CSS to apply different styles based on the screen size. React allows developers to dynamically apply CSS classes based on the screen size, making it easier to create responsive designs.
Another technique is using React’s built-in features such as flexbox and grid to create flexible layouts that adapt to different screen sizes. These features allow developers to create responsive designs without the need for complex CSS calculations.
In addition to responsiveness, user-friendliness is also important in web applications. React provides several best practices for designing and developing user interfaces. These include using clear and concise labels, providing feedback to users, and ensuring accessibility for users with disabilities.
By following these best practices, React developers can create web applications that are not only visually appealing but also responsive and user-friendly.
Debugging and Troubleshooting React Applications
Like any software development project, React applications can encounter issues that need to be debugged and troubleshooted. Common issues faced by React developers include rendering errors, state management issues, and performance problems.
To debug React applications, developers can use browser developer tools such as Chrome DevTools or Firefox Developer Tools. These tools allow developers to inspect the DOM, view console logs, and debug JavaScript code. By using these tools, developers can identify and fix issues in their React applications.
In addition to browser developer tools, React developers can also use React-specific debugging tools such as React DevTools. These tools provide additional features specifically designed for debugging React applications, such as inspecting component hierarchies and viewing component props and state.
To troubleshoot performance issues, React developers can use performance profiling tools such as React Profiler. These tools allow developers to identify performance bottlenecks in their applications and optimize them for better performance.
By using these debugging and troubleshooting techniques, React developers can ensure that their applications are free from errors and perform optimally.
Staying Up-to-Date with the Latest React Trends and Technologies
Staying up-to-date with the latest React trends and technologies is crucial for a React developer to stay competitive in the industry. React is constantly evolving, with new features and improvements being released regularly.
To stay up-to-date with React, developers can follow blogs and websites dedicated to React development. These resources provide tutorials, articles, and updates on the latest trends and technologies in the React ecosystem.
In addition to blogs and websites, attending conferences and meetups is also a great way to stay updated with the latest React trends. These events often feature talks and workshops by industry experts who share their knowledge and insights on React development.
Implementing new React technologies and features requires careful consideration and planning. It is important for developers to thoroughly understand the new technology or feature before implementing it in their projects. They should also consider the impact on performance, compatibility with existing codebase, and potential benefits before adopting new technologies or features.
By staying up-to-date with the latest React trends and technologies, developers can ensure that they are using the best tools and techniques to build high-quality web applications.
Working in Agile and Scrum Environments
Agile and Scrum methodologies are widely used in the software development industry, including web development. Agile is an iterative and incremental approach to software development, while Scrum is a specific framework within the Agile methodology.
Working in Agile and Scrum environments is important for a React developer as it promotes collaboration, flexibility, and adaptability. In Agile and Scrum, development is done in short iterations called sprints, usually lasting 1-2 weeks. This allows for frequent feedback and iteration, resulting in faster delivery of high-quality software.
As a React developer, it is important to actively participate in Agile ceremonies such as daily stand-ups, sprint planning, and retrospectives. These ceremonies provide opportunities to communicate progress, discuss challenges, and plan for the next sprint.
In addition to participating in Agile ceremonies, React developers should also embrace the Agile mindset of continuous improvement. They should be open to feedback, willing to adapt to changing requirements, and constantly looking for ways to improve their skills and processes.
By working in Agile and Scrum environments, React developers can contribute to the success of their projects by delivering high-quality software in a timely manner.
Career Growth Opportunities for React Developers
React developers have numerous career growth opportunities within the web development field. As they gain experience and expertise in React, they can advance within their web development team to more senior roles such as lead developer or technical architect.
In addition to advancement within a web development team, React developers can also branch out into related fields such as mobile app development or software engineering. React Native, a framework for building native mobile apps using React, allows React developers to leverage their existing skills and knowledge to build mobile applications.
Furthermore, with the increasing demand for web applications and the continuous evolution of technology, there are ample opportunities for React developers to work on exciting projects and contribute to innovative solutions.
In conclusion, React development plays a crucial role in building modern web applications. To become a successful React developer, one must possess the essential technical skills and qualifications, as well as soft skills such as communication and problem-solving. The role of a React developer involves building reusable components, integrating with backend APIs, and testing and debugging applications. Collaboration with designers and backend developers is important for a React developer to ensure a seamless development process. Building responsive and user-friendly web applications is essential in today’s digital landscape, and React provides the necessary tools and techniques to achieve this. Staying up-to-date with the latest React trends and technologies is crucial for a React developer to stay competitive in the industry. Working in Agile and Scrum environments promotes collaboration and adaptability, which are important qualities for a React developer. Finally, React developers have numerous career growth opportunities within the web development field, as well as opportunities to branch out into related fields such as mobile app development or software engineering.
The Thriving React Developer Job Market: Opportunities and Trends
React is a popular JavaScript library that is widely used in web development. It was developed by Facebook and has gained immense popularity due to its simplicity, flexibility, and efficiency. React allows developers to build user interfaces for web applications by creating reusable UI components. As a result, React has become the go-to choice for many developers and companies in the industry.
With the increasing demand for web applications, the job market for React developers has also seen significant growth. Companies are actively seeking skilled React developers to build and maintain their web applications. This has created a high demand for professionals who are proficient in React and have the ability to create efficient and scalable web applications.
Key Takeaways
- React developers are in high demand in the job market due to the popularity of the React library.
- React developers have opportunities in various industries, including e-commerce, healthcare, and finance.
- Trends in React development include server-side rendering, progressive web apps, and virtual reality.
- React skills are important for web development because of its component-based architecture and efficient rendering.
- React developer salaries can range from ,000 to 0,000 depending on experience and location.
Why React Developers are in High Demand
There are several reasons why React developers are in high demand in the job market. Firstly, React offers numerous benefits for web development. It allows developers to create reusable UI components, which makes it easier to maintain and update web applications. This saves time and effort, making React a preferred choice for many developers.
Secondly, React is highly efficient and performs well even with large-scale applications. It uses a virtual DOM (Document Object Model) which allows it to update only the necessary components when changes occur, resulting in faster rendering and improved performance.
Lastly, React has a large and active community of developers who contribute to its growth and development. This means that there is a wealth of resources, tutorials, and libraries available for React developers, making it easier to learn and master the technology.
Opportunities for React Developers in Various Industries
React developers have opportunities in various industries due to the widespread adoption of React in web development. Industries such as e-commerce, finance, healthcare, and technology are actively hiring React developers to build their web applications.
For example, companies like Amazon, Netflix, Airbnb, and Facebook are using React in their web development projects. These companies have a high demand for skilled React developers who can create efficient and user-friendly web applications.
In addition, startups and small businesses are also hiring React developers to build their web applications. The flexibility and scalability of React make it an ideal choice for startups that are looking to quickly develop and launch their products.
Trends in React Development: What’s New and What’s Next
Trend | Description | Impact |
---|---|---|
React Native | Developing mobile apps using React | Increased efficiency and cost-effectiveness in mobile app development |
Server-Side Rendering | Rendering React components on the server | Improved performance and SEO for web applications |
GraphQL | Query language for APIs | Efficient data fetching and reduced network traffic |
React Hooks | New way of using state and lifecycle methods | Improved code readability and reduced complexity |
React 18 | Upcoming release with new features and improvements | Enhanced developer experience and performance |
React development is constantly evolving, with new trends and features being introduced regularly. One of the latest trends in React development is the use of server-side rendering (SSR). SSR allows the initial rendering of a web page to be done on the server, which improves performance and SEO (Search Engine Optimization).
Another trend in React development is the use of state management libraries such as Redux and Mob
These libraries help manage the state of an application, making it easier to handle complex data flows and improve performance.
React also continues to release new features and updates to improve developer experience and performance. Some of the recent updates include the introduction of React Hooks, which allow developers to use state and other React features without writing a class component.
The Importance of React Skills for Web Development
Having React skills is essential for web development due to its widespread adoption and popularity in the industry. React is used by many companies to build their web applications, so having React skills can significantly increase your job prospects.
One advantage of having React skills is that it makes you a more versatile developer. React can be used with other technologies such as Node.js, GraphQL, and Redux, so having knowledge of these technologies along with React can make you a valuable asset to any development team.
In addition, React skills are highly transferable. Once you have mastered React, it becomes easier to learn other JavaScript frameworks such as Angular or Vue.js. This means that even if the job market shifts towards another framework in the future, your React skills will still be valuable.
React Developer Salaries: How Much Can You Make?
React developers are in high demand, which means that they can command competitive salaries in the job market. According to various salary reports, the average salary for React developers ranges from $80,000 to $120,000 per year, depending on factors such as experience, location, and company size.
Factors that can affect React developer salaries include the level of experience. Junior React developers with less than two years of experience can expect to earn an average salary of around $70,000 per year. Mid-level React developers with two to five years of experience can earn an average salary of around $90,000 per year. Senior React developers with more than five years of experience can earn an average salary of over $100,000 per year.
Location is another factor that can affect React developer salaries. Salaries tend to be higher in tech hubs such as San Francisco, New York City, and Seattle, where the cost of living is higher. However, even in smaller cities or remote positions, React developers can still earn competitive salaries.
Top Companies Hiring React Developers Today
Many top companies are actively hiring React developers today. These companies recognize the value of React in building efficient and scalable web applications. Some of the top companies that are currently hiring React developers include:
1. Amazon: Amazon uses React for its e-commerce platform and is constantly looking for skilled React developers to improve its user interface and customer experience.
2. Netflix: Netflix uses React for its streaming platform and is always looking for talented React developers to enhance its user interface and optimize performance.
3. Facebook: As the creator of React, Facebook is a major employer of React developers. They are constantly looking for skilled developers to work on their various web applications.
4. Airbnb: Airbnb uses React for its web application and is actively hiring React developers to improve its user interface and user experience.
5. Google: Google uses React for its web applications and is always looking for talented React developers to work on its various projects.
Tips for Landing Your Dream React Developer Job
To land your dream React developer job, it is important to prepare and stand out from other candidates. Here are some tips to help you succeed:
1. Build a strong portfolio: Showcase your React skills by building projects and creating a portfolio that demonstrates your abilities. Include links to your GitHub or other repositories where potential employers can see your code.
2. Stay up-to-date with the latest trends and updates in React: Keep learning and improving your React skills by staying updated with the latest trends, features, and updates in React development. This will show potential employers that you are dedicated to your craft.
3. Network and attend industry events: Attend meetups, conferences, and other industry events to network with other developers and potential employers. This can help you make connections and learn about job opportunities.
4. Prepare for technical interviews: Be prepared for technical interviews by practicing coding exercises and familiarizing yourself with common interview questions for React developers. This will help you feel more confident during the interview process.
5. Showcase your problem-solving skills: During interviews, emphasize your problem-solving skills and ability to think critically. Employers are looking for developers who can solve complex problems and come up with innovative solutions.
The Future of React Development: Predictions and Prospects
The future of React development looks promising, with continued growth and adoption in the industry. As web applications become more complex and demanding, the need for efficient and scalable frameworks like React will continue to rise.
One of the potential opportunities for React developers in the future is the growth of mobile app development using React Native. React Native allows developers to build native mobile apps using JavaScript and React, which makes it easier to develop cross-platform applications.
Another potential opportunity for React developers is the rise of serverless architecture. Serverless architecture allows developers to build applications without managing servers, which can improve scalability and reduce costs. React can be used in conjunction with serverless technologies such as AWS Lambda or Firebase to build serverless web applications.
However, there are also challenges that React developers may face in the future. As React continues to evolve, developers will need to keep up with the latest updates and features. This requires continuous learning and staying updated with the latest trends in React development.
How to Thrive in the React Developer Job Market
In conclusion, the job market for React developers is thriving due to the popularity and benefits of React in web development. React developers are in high demand in various industries, and there are numerous opportunities for growth and advancement.
To thrive in the React developer job market, it is important to continuously improve your skills, stay updated with the latest trends, and build a strong portfolio. Networking and attending industry events can also help you make connections and learn about job opportunities.
By following these tips and staying dedicated to your craft, you can succeed as a React developer in the job market and build a rewarding career in web development.
Shopify Store Development: Crafting User-Friendly E-commerce Experiences
Hey there, readers! Maya Anderson here, coming at you with some real talk about Shopify store development. You know, it’s kinda like building a house. You want it to not only look snazzy but also be comfy and easy for folks to move around in. That’s what we aim for in e-commerce: a slick, user-friendly experience that keeps customers coming back.
The Starting Line: Where It All Begins
So, I remember this one project we had at Modern Labyrinth. Man, it was a doozy! Our client, let’s call her Jane, was all over the place with her ideas. She wanted to sell handmade soaps that smelled like different cities around the world. Pretty neat, right? But her existing site? It was a hot mess. Slow as molasses and as confusing as a corn maze.
The Power of Shopify
First things first, why Shopify? It’s the Swiss Army knife of e-commerce platforms, folks. It’s user-friendly, versatile, and it’s got all these nifty tools that make selling online as easy as pie. Plus, it scales with your business – like yoga pants, it stretches to fit!
Design: More Than Just a Pretty Face
Now, let’s chat about design. You wouldn’t wear socks with sandals, right? Same deal here – your store’s gotta have style AND substance. It’s about creating a vibe that resonates with your audience. Jane’s soaps? We gave them a travel-themed design, like a mini vacation in a bar of soap.
Here’s a fun fact: did you know goldfish have longer attention spans than humans now? Yep, you read that right. So, your site needs to grab attention fast. Make it intuitive. Jane’s site? We streamlined that baby so you could find your Parisian Lavender or Tokyo Cherry Blossom soap in two clicks, tops.
The Techy Stuff: Optimization and Functionality
Alright, let’s geek out for a sec. Optimizing a Shopify store isn’t just about making it look good. It’s about making it run smoother than a fresh jar of Skippy. We’re talking mobile optimization, fast load times, and seamless checkout processes.
In the world of e-commerce, if you snooze, you lose. Staying updated with the latest trends is key. Augmented reality, voice search, AI chatbots – it’s all fair game. For Jane’s store, we integrated a cool AR feature. Wanna see how that soap would look in your bathroom? Just point your phone and bam!
Let’s not beat around the bush – the goal is to make sales. But it’s more than that. It’s about creating an experience that makes customers feel good. When they’re happy, they come back, and they tell their friends. That’s the real magic sauce, y’all.
Overall, It’s a Wrap!
In closing, Shopify store development? It’s a wild ride, but oh so worth it. Thanks for hanging with me on this little journey. Keep aiming for those stars, and remember, in the world of e-commerce, the sky’s the limit!
WordPress and WooCommerce: Revolutionizing E-Commerce for Online Retail Success
Hey there, Maya here! So, I’ve been noodling around with WordPress and WooCommerce at Modern Labyrinth, and lemme tell ya, it’s been quite the ride. Got some thoughts and stories to share with y’all, so buckle up!
WooCommerce: The Unsung Hero of E-Commerce
Now, onto WooCommerce. This gem is like the secret sauce to your favorite burger – it just makes everything better. It turns your WordPress site into a fully-fledged online store. We’ve been integrating it for clients, and the results? Stellar! Sales graphs going up like Elon Musk’s rockets.
Personal Anecdote: A WooCommerce Win
So, there was this one time, we were setting up a store for a client selling handmade soaps. We added some cool WooCommerce features, and guess what? Their sales doubled in just a month! Talk about a eureka moment!
WooCommerce Plugins: The Game Changers
WooCommerce plugins are like those little Lego pieces that make your model go from “meh” to “wow.” From payment gateways to inventory management, these plugins make everything a breeze. Plus, they’re always updating with new stuff – it’s like Christmas every day!
Challenges and Triumphs
Okay, it hasn’t always been smooth sailing. There were times when a plugin update felt like a jump scare in a horror movie. But hey, that’s where problem-solving kicks in. And overcoming these challenges? Super satisfying!
The Future of E-Commerce with WordPress and WooCommerce
The future’s looking bright, folks. With WordPress and WooCommerce, the possibilities are endless. It’s like we’re on the Starship Enterprise, heading to new frontiers in the e-commerce universe.
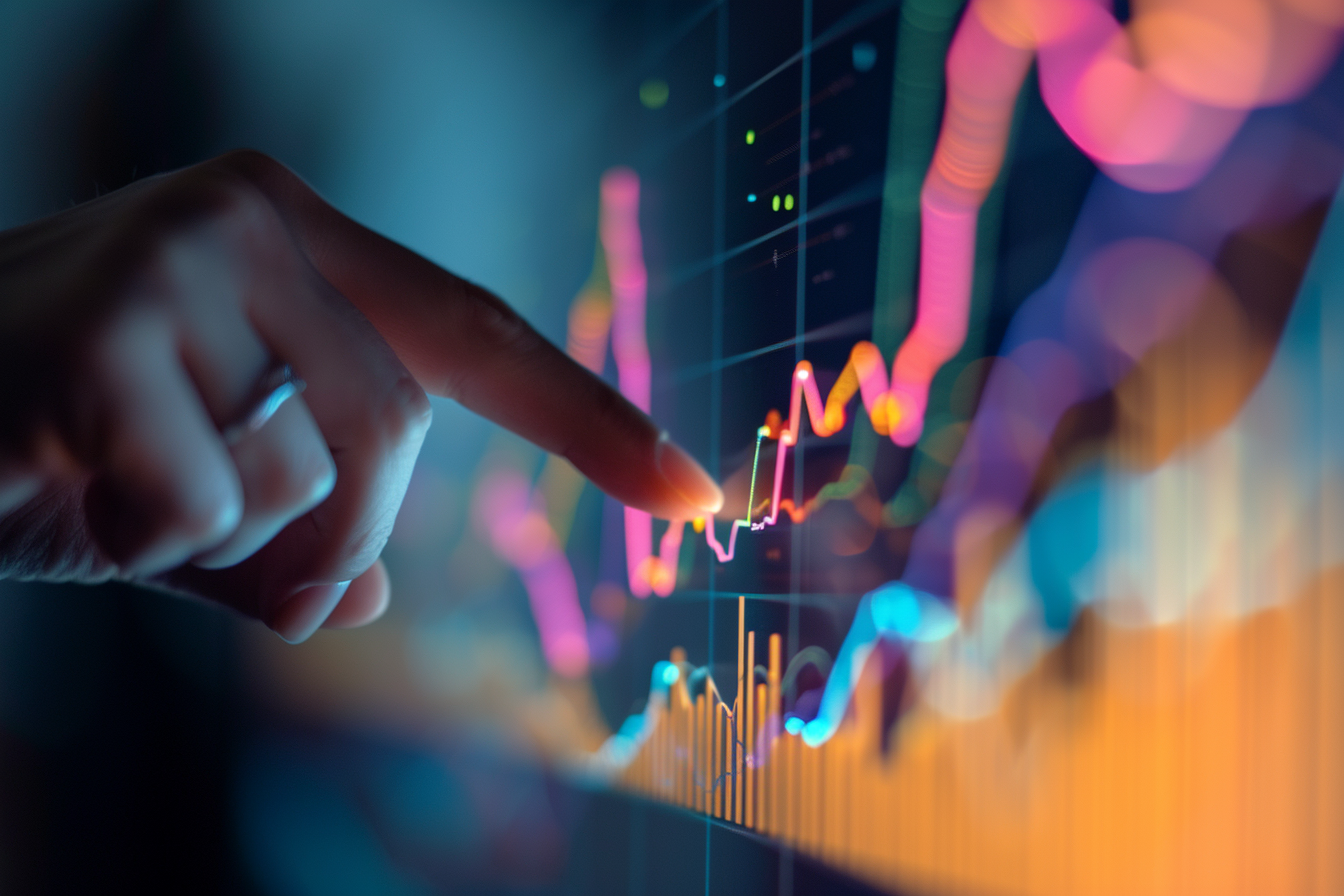
Final Thoughts
Overall, WordPress and WooCommerce are like peanut butter and jelly – perfect together. They’re revolutionizing e-commerce, making it accessible and efficient for everyone. Can’t wait to see what’s next!
In closing, thanks for reading, and keep surfing the tech wave! And remember, in the world of web development, always stay curious!
Navigating the Future: Modern Labyrinth’s Role in Web Application Evolution
Hey there! I’m Maya, a techie at heart and a web dev enthusiast at Modern Labyrinth. So, have you ever wondered how these web apps have transformed over the years? It’s like, one day we’re playing Snake on our Nokia phones, and the next thing you know, we’re controlling our entire homes from our smartphones!
When I joined Modern Labyrinth a couple of years ago, it was clear that web apps were more than just digital tools—they’re the canvas where technology meets creativity. My journey started with basic HTML (oh, the good ol’ days!), but as the tech world evolved, so did my skills.
Riding the Wave of Change: Our Journey
We’ve seen it all at Modern Labyrinth. Remember the days when websites were just digital brochures? Well, not anymore! Web apps today are smarter, faster, and more intuitive. It’s like they know what you want before you do. And guess what? We’re at the forefront of this change.
We’re not just coding; we’re crafting experiences. My team and I dive deep into JavaScript frameworks, dance with APIs, and play with cloud services. And it’s not always smooth sailing—deadlines, bugs, and the occasional coffee spill on the keyboard (oops!). But hey, that’s the life of a web dev, right?
A Peek Behind the Curtain: Our Secret Sauce
So, what’s our secret at Modern Labyrinth? It’s simple, yet profound: User-first, innovation always. We’re not just building apps; we’re building relationships. It’s all about creating that ‘wow’ moment for our users.
We experiment, we fail, we learn, and we grow. That’s our mantra. Whether it’s optimizing load times or creating seamless user interfaces, we’re always on our toes. And let me tell you, there’s nothing more thrilling than seeing your code come to life and solve real-world problems.
Where We’re Headed: The Future of Web Apps
Looking ahead, the possibilities are endless. Artificial intelligence, virtual reality, quantum computing—you name it, we’re exploring it. It’s not just about staying ahead of the curve; it’s about bending it.
At Modern Labyrinth, we’re not just developers; we’re dreamers. We’re shaping a future where web apps are not just part of our lives but an extension of ourselves. It’s a journey, and I’m so stoked to be a part of it.
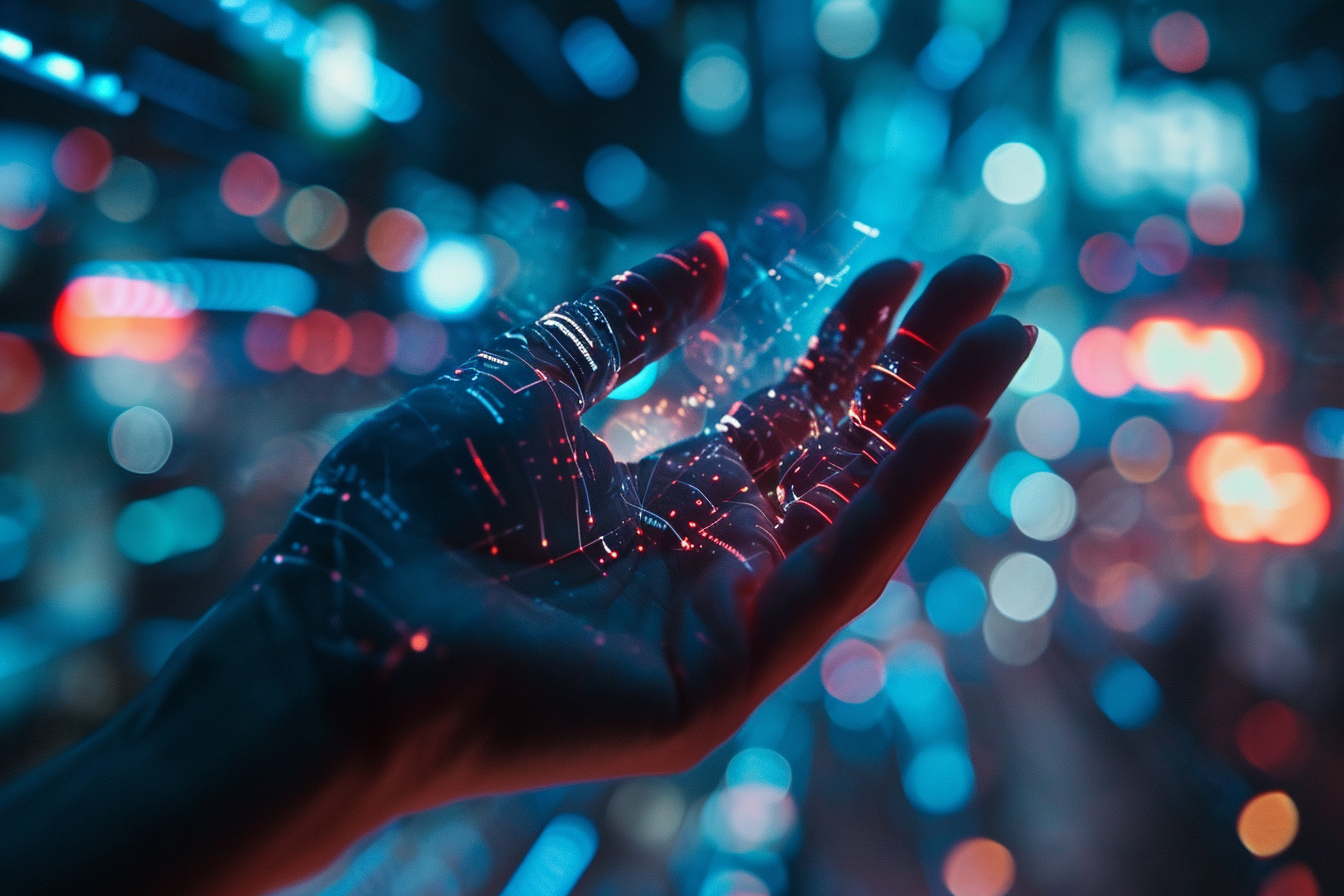
In Closing…
Overall, it’s been an amazing ride with Modern Labyrinth. Every day is a new challenge, a new opportunity to push the boundaries. And to all the aspiring web devs out there, keep coding, keep dreaming. The digital world is your oyster!
Thanks for reading, folks! Stay curious, stay innovative.