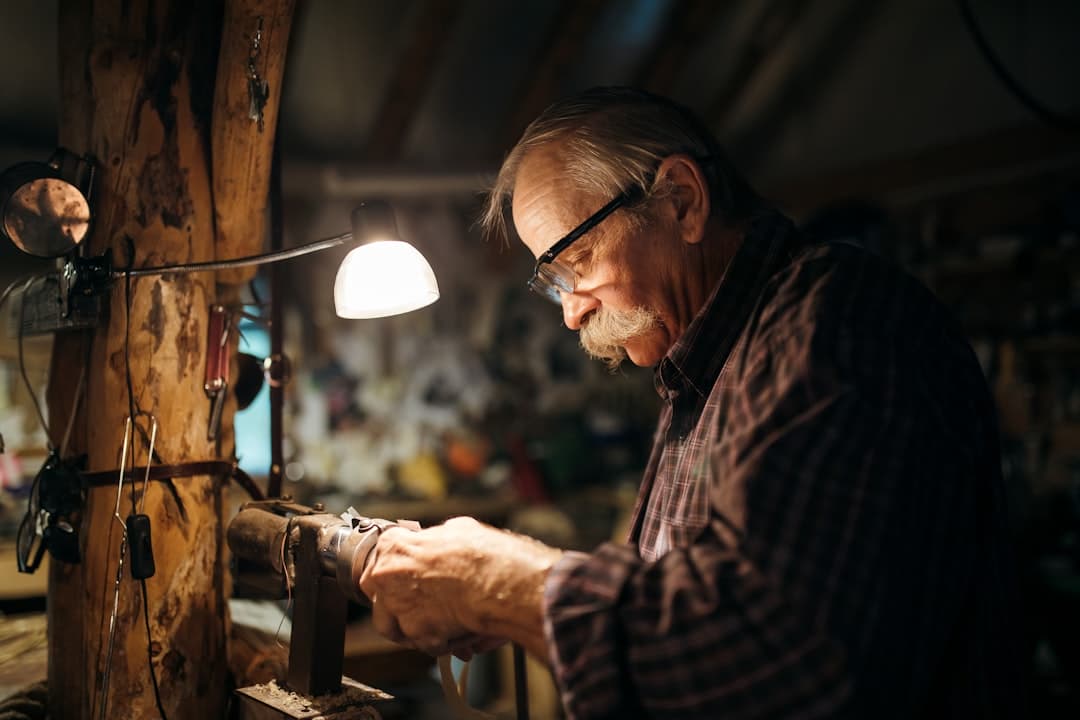
React Developer Tools is a browser extension that allows developers to inspect and debug React components in their applications. It provides a set of tools that make it easier to understand and manipulate the component hierarchy, inspect component properties and state, and optimize performance. React Developer Tools is available for Chrome, Firefox, and Edge browsers, and it is an essential tool for any React developer.
One of the main benefits of using React Developer Tools is that it provides a visual representation of the component hierarchy, also known as the React Component Tree. This allows developers to easily navigate through the different components in their application and understand how they are structured. It also helps in identifying any issues or bugs in the component hierarchy, making it easier to debug and fix them.
Key Takeaways
- React Developer Tools is a browser extension that helps developers debug and optimize React applications.
- Installing React Developer Tools is easy and can be done through the Chrome or Firefox extension stores.
- The React Component Tree allows developers to visualize the hierarchy of components in their application.
- Inspecting component properties and state can help developers identify and fix bugs in their code.
- React Developer Tools can also be used to optimize performance by identifying and addressing performance bottlenecks.
Installing React Developer Tools
Installing React Developer Tools is a straightforward process. Here is a step-by-step guide on how to install it:
1. Open your preferred browser (Chrome, Firefox, or Edge).
2. Go to the browser’s extension store (Chrome Web Store, Firefox Add-ons, or Microsoft Edge Add-ons).
3. Search for “React Developer Tools” in the extension store.
4. Click on the “Add to Chrome/Firefox/Edge” button.
5. Confirm the installation by clicking on “Add extension” or similar.
6. Once installed, you should see the React Developer Tools icon in your browser’s toolbar.
Alternatively, you can also install React Developer Tools from the official website (https://reactjs.org/blog/2019/08/15/new-react-devtools.html). Simply download the extension file for your browser and follow the installation instructions provided.
Exploring the React Component Tree
The React Component Tree is a hierarchical structure that represents the relationship between different components in a React application. It starts with the root component and branches out to child components, forming a tree-like structure.
With React Developer Tools, you can easily explore the React Component Tree of your application. Simply open the developer tools panel in your browser and navigate to the “Components” tab. Here, you will see a visual representation of the component hierarchy.
You can expand and collapse different components to view their child components. This helps in understanding how the components are nested and how they interact with each other. You can also inspect the props and state of each component, which we will discuss in the next section.
Inspecting React Component Properties and State
Property/State | Description | Type | Default Value |
---|---|---|---|
props | The properties passed to the component | Object | {} |
state | The internal state of the component | Object | {} |
componentDidMount() | Method called after the component is mounted | Function | – |
componentDidUpdate(prevProps, prevState) | Method called after the component is updated | Function | – |
shouldComponentUpdate(nextProps, nextState) | Method that determines if the component should update | Function | true |
React components have properties (props) and state, which determine their behavior and appearance. React Developer Tools allows you to inspect these props and state values, making it easier to understand how a component is functioning.
To inspect the props and state of a component, simply select the component in the React Component Tree view. In the right-hand panel, you will see two tabs: “Props” and “State”. Clicking on these tabs will display the corresponding values for the selected component.
The “Props” tab shows the props passed to the component, including their names and values. This is useful for understanding how data is being passed down from parent components to child components.
The “State” tab shows the current state of the component. It displays all the state variables defined in the component, along with their current values. This is helpful for debugging and understanding how the component’s state changes over time.
Debugging React Components with React Developer Tools
React Developer Tools provides powerful debugging capabilities that can help identify and fix issues in React components. Here are some techniques for debugging React components using React Developer Tools:
1. Setting breakpoints: You can set breakpoints in your code directly from the React Component Tree view. Simply right-click on a component and select “Break on > All Updates” or “Break on > Render”. This will pause the execution of your code whenever that component is updated or rendered, allowing you to inspect the component’s props and state at that point in time.
2. Inspecting component updates: React Developer Tools allows you to track component updates and see what triggered them. In the “Components” tab, you can enable the “Highlight Updates” option, which will highlight components that have been updated since the last render. This can help identify unnecessary re-renders and optimize performance.
3. Console logging: You can log messages to the browser console directly from React Developer Tools. Simply select a component in the React Component Tree view, right-click, and choose “Log Component”. This will log the selected component to the console, along with its props and state. You can also log specific values or expressions by selecting them in the “Props” or “State” tabs and choosing “Log Value”.
Using React Developer Tools to Optimize Performance
React Developer Tools provides several features that can help optimize the performance of your React application. Here are some techniques for using React Developer Tools to optimize performance:
1. Profiling: React Developer Tools includes a built-in profiler that allows you to measure the performance of your components. To start profiling, click on the “Profiler” tab in the developer tools panel. Then, interact with your application to trigger different actions or events. The profiler will record the time taken by each component to render and update. This information can be used to identify performance bottlenecks and optimize slow components.
2. Highlighting unnecessary re-renders: As mentioned earlier, React Developer Tools can highlight components that have been updated since the last render. This can help identify unnecessary re-renders caused by inefficient code or unnecessary prop changes. By optimizing these re-renders, you can improve the overall performance of your application.
3. Analyzing component dependencies: React Developer Tools allows you to analyze the dependencies between components in your application. By selecting a component in the React Component Tree view and clicking on the “Dependencies” tab, you can see which components are consuming its props. This can help identify components that are unnecessarily re-rendering due to changes in their dependencies. By optimizing these dependencies, you can reduce the number of re-renders and improve performance.
Debugging React Native Applications with React Developer Tools
React Developer Tools can also be used to debug React Native applications. The process is similar to debugging web applications, but with a few additional steps.
To debug a React Native application using React Developer Tools, follow these steps:
1. Enable remote debugging in your React Native application by adding the following line of code to your entry file (usually `index.js` or `App.js`):
“`javascript
import { AppRegistry } from ‘react-native’;
import App from ‘./App’;
AppRegistry.registerComponent(‘YourAppName’, () => App);
AppRegistry.runApplication(‘YourAppName’, {
rootTag: document.getElementById(‘root’),
});
“`
2. Open your React Native application in a simulator or on a physical device.
3. Open the developer tools panel in your browser and navigate to the “React” tab.
4. Click on the “Select a view” button and choose your React Native application from the list.
5. You should now see the React Component Tree and other debugging features specific to React Native.
Advanced Features of React Developer Tools
In addition to the basic features we have discussed so far, React Developer Tools also offers some advanced features that can further enhance your development workflow. Here are a few examples:
1. Time-travel debugging: React Developer Tools allows you to time-travel through the state and props changes of your components. This can be useful for understanding how the component’s state evolves over time and for reproducing specific scenarios or bugs.
2. Editing component props and state: With React Developer Tools, you can edit the props and state of a component directly from the developer tools panel. This can be helpful for testing different scenarios or making quick changes to the component’s behavior.
3. Exporting and importing component snapshots: React Developer Tools allows you to export and import snapshots of your components. This can be useful for sharing component states or for saving and restoring specific application states during development.
Tips and Tricks for Using React Developer Tools
Here are some tips and tricks for using React Developer Tools more efficiently:
1. Use keyboard shortcuts: React Developer Tools provides several keyboard shortcuts that can speed up your workflow. For example, you can press “Ctrl + P” (or “Cmd + P” on Mac) to quickly search for a specific component in the React Component Tree view.
2. Customize the display: You can customize the display of React Developer Tools to suit your preferences. For example, you can change the theme, adjust the font size, or hide certain panels that you don’t use frequently.
3. Use the “Inspect” mode: React Developer Tools includes an “Inspect” mode that allows you to select a component directly from your application’s U
Simply right-click on an element in your application and choose “Inspect” to select the corresponding component in the React Component Tree view.
Conclusion and Next Steps for Improving Your React Development Workflow
In conclusion, React Developer Tools is a powerful tool that provides a wide range of features for inspecting, debugging, and optimizing React components. By using React Developer Tools, you can gain a deeper understanding of your application’s component hierarchy, inspect component properties and state, debug issues more effectively, optimize performance, and even debug React Native applications.
To further improve your React development workflow, consider exploring more advanced features of React Developer Tools, experimenting with different debugging techniques, and staying up-to-date with the latest updates and improvements in the tool. By leveraging the full potential of React Developer Tools, you can become a more efficient and effective React developer.
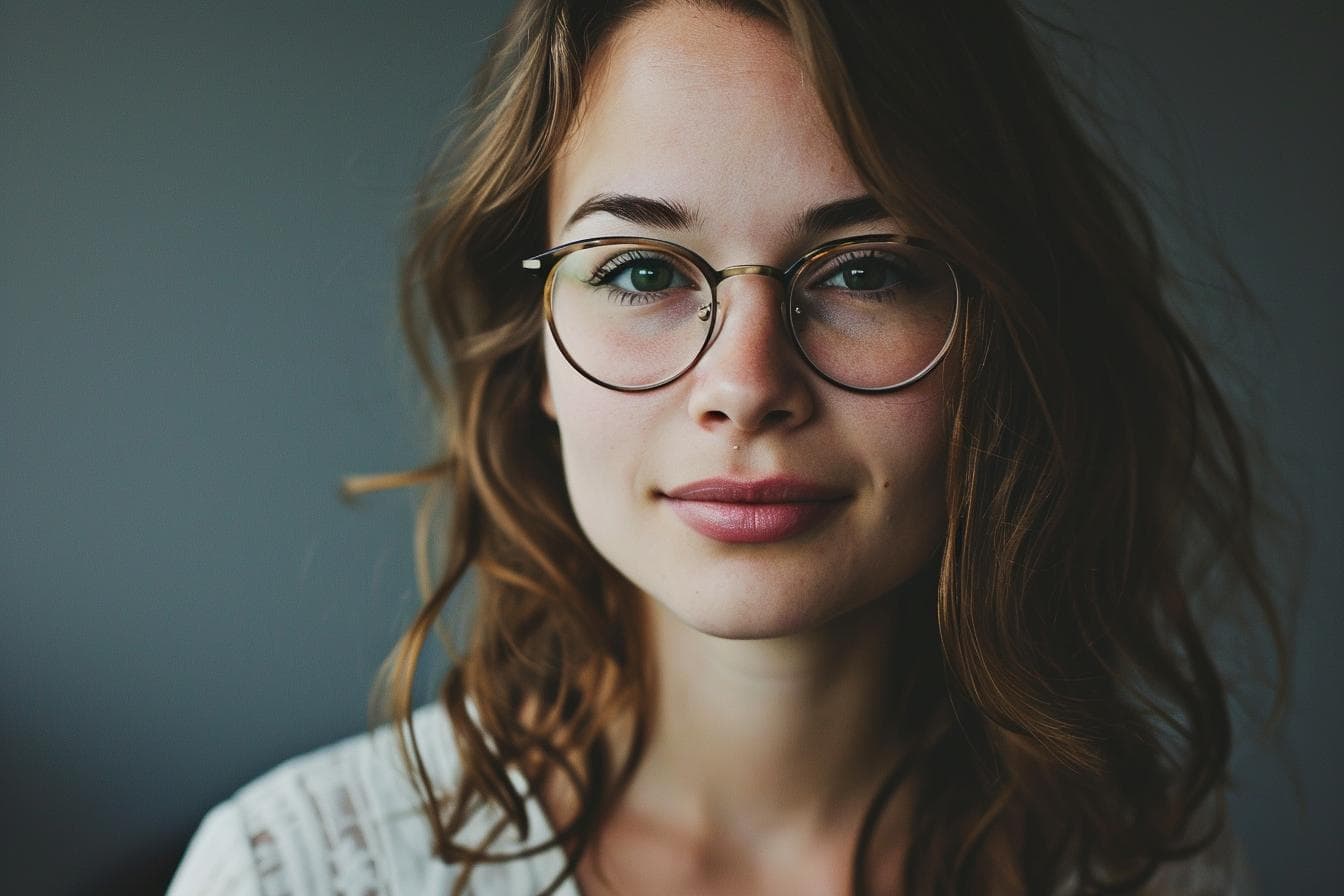