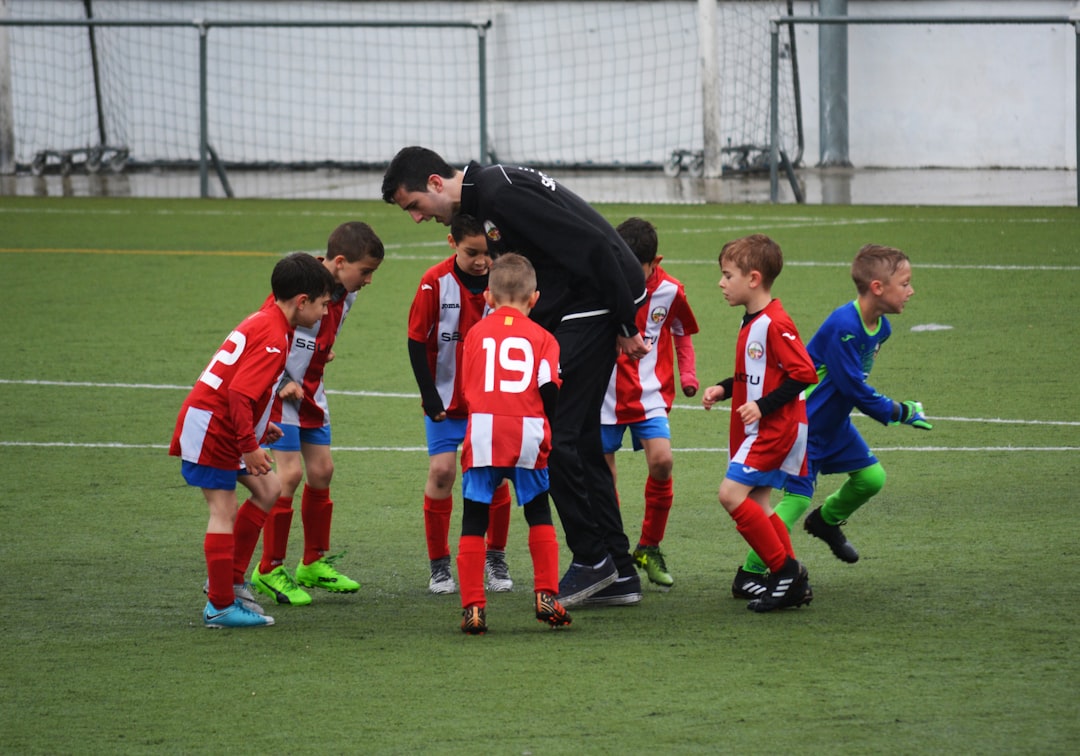
React is a popular JavaScript library for building user interfaces. It was developed by Facebook and has gained immense popularity in the web development community. React allows developers to build reusable UI components that can be easily composed to create complex user interfaces. One of the key features of React is its virtual DOM, which allows for efficient rendering and updating of components.
React’s popularity can be attributed to its simplicity and flexibility. It provides a declarative syntax for defining UI components, making it easier to understand and maintain code. React also encourages the use of reusable components, which can save developers time and effort when building applications.
In addition to its core library, React has a vast ecosystem of tools and libraries that enhance its functionality. These include state management libraries like Redux and MobX, routing libraries like React Router, and testing frameworks like Jest. These tools make it easier for developers to build complex applications with React.
Key Takeaways
- React is a popular JavaScript library for building user interfaces.
- React components are reusable building blocks that can be composed to create complex UIs.
- React state management allows for dynamic updates to the UI based on user interactions or data changes.
- React routing and navigation enable users to move between different pages or views within a single-page application.
- React hooks and context API provide a way to manage state and share data between components without using class components.
Understanding React Components
In React, components are the building blocks of user interfaces. They are reusable pieces of code that encapsulate the logic and presentation of a part of the U
Components can be either functional or class-based.
Functional components are simple JavaScript functions that return JSX (JavaScript XML), which is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. Functional components are easier to read and test, and they are recommended for most use cases.
Class components are ES6 classes that extend the React.Component class. They have additional features like lifecycle methods and access to state and props. Class components are useful when you need to manage state or use lifecycle methods.
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. It is used to define the structure and appearance of React components. JSX makes it easier to write and understand code by combining HTML-like syntax with JavaScript logic.
React State Management
State Management Library | Popularity | Learning Curve | Performance |
---|---|---|---|
Redux | Very popular | Steep | High performance |
MobX | Popular | Easy | High performance |
Context API | Increasingly popular | Easy | Medium performance |
State is an important concept in React. It represents the data that a component needs to render and update itself. State is mutable and can be changed using the setState method.
The setState method is used to update the state of a component. It takes an object as an argument, which contains the new values for the state properties. When setState is called, React re-renders the component with the updated state.
In addition to the built-in state management in React, there are also state management libraries like Redux and Mob
These libraries provide a centralized way to manage state in larger applications. They allow you to define actions and reducers to update and retrieve state, making it easier to manage complex application state.
React Routing and Navigation
Routing and navigation are important aspects of building web applications. In React, routing is handled by a library called React Router. React Router provides a declarative way to define routes and navigate between them.
React Router allows you to define routes using the Route component. Each route is associated with a specific component that will be rendered when the route matches the current URL. React Router also provides features like nested routes, dynamic routing, and route parameters.
Nested routes allow you to define routes within other routes. This is useful when you have components that need to be rendered within a parent component. Dynamic routing allows you to define routes with dynamic segments, which can be used to pass data or parameters to the rendered component.
React Hooks and Context API
React Hooks are a new feature introduced in React 16.8 that allows you to use state and other React features without writing class components. Hooks provide a simpler and more intuitive way to manage state and side effects in functional components.
The useState hook allows you to add state to functional components. It takes an initial value as an argument and returns an array with two elements: the current state value and a function to update the state.
The useEffect hook allows you to perform side effects in functional components. Side effects include things like fetching data, subscribing to events, or manually changing the DOM. The useEffect hook takes a function as an argument and runs it after every render.
The useContext hook allows you to access the value of a context directly in a functional component. The Context API is a feature of React that allows you to share data between components without passing props manually.
Advanced React Concepts
In addition to the basic concepts of React, there are also more advanced concepts that can be used to build more complex applications.
Server-side rendering (SSR) is a technique that allows you to render React components on the server and send the HTML to the client. This can improve performance and SEO, as the initial page load is faster and search engines can index the content.
Code splitting is a technique that allows you to split your code into smaller chunks and load them on demand. This can improve performance by reducing the initial load time of your application.
Lazy loading is a technique that allows you to load components or resources only when they are needed. This can improve performance by reducing the amount of code and resources that need to be loaded initially.
HOCs (Higher-Order Components) are functions that take a component as an argument and return a new component with additional functionality. HOCs are useful for adding common functionality to multiple components.
Render props is a pattern where a component accepts a function as a prop and calls it with its internal state or other data. This allows for greater flexibility and reusability of components.
React Native is a framework for building mobile applications using React. It allows you to write code once and deploy it on multiple platforms, including iOS and Android.
Building Real-world React Applications
Building real-world applications with React involves understanding common application architectures and patterns.
One common architecture is the component-based architecture, where the UI is divided into reusable components. This allows for easier maintenance and reusability of code.
Another common pattern is the container/component pattern, where container components are responsible for fetching data and managing state, while presentational components are responsible for rendering the U
There are also popular React frameworks like Next.js and Gatsby that provide additional features and functionality for building real-world applications. Next.js is a framework for server-rendered React applications, while Gatsby is a framework for building static websites.
Testing and Debugging React Applications
Testing and debugging are important aspects of building reliable and bug-free applications.
Jest is a popular testing framework for React applications. It provides a simple and intuitive API for writing tests and comes with built-in support for mocking and code coverage.
Enzyme is a testing utility for React that provides a set of APIs for interacting with React components. It allows you to simulate events, query the rendered output, and assert on the component’s state and props.
React Developer Tools is a browser extension that allows you to inspect and debug React components in the browser. It provides a tree view of the component hierarchy, as well as tools for inspecting props, state, and performance.
Chrome DevTools is a set of web developer tools built into the Chrome browser. It provides tools for debugging JavaScript, inspecting network requests, profiling performance, and more.
Optimizing React Performance
Performance optimization is important to ensure that your React application runs smoothly and efficiently.
React Profiler is a tool that allows you to measure the performance of your React components. It provides insights into how long each component takes to render, as well as how often they are rendered.
There are also performance monitoring tools like New Relic and Datadog that can help you identify performance bottlenecks in your application. These tools provide real-time monitoring of your application’s performance metrics, allowing you to identify and fix issues quickly.
Deploying React Applications to Production
Deploying a React application to production involves hosting the application on a server and making it accessible to users.
There are popular hosting platforms like Netlify and Heroku that provide easy deployment options for React applications. These platforms allow you to deploy your application with a single command and provide features like automatic SSL, CDN, and continuous deployment.
CI/CD (Continuous Integration/Continuous Deployment) pipelines are a set of practices and tools that automate the process of building, testing, and deploying software. They allow you to automate the deployment of your React application, ensuring that it is always up-to-date and bug-free.
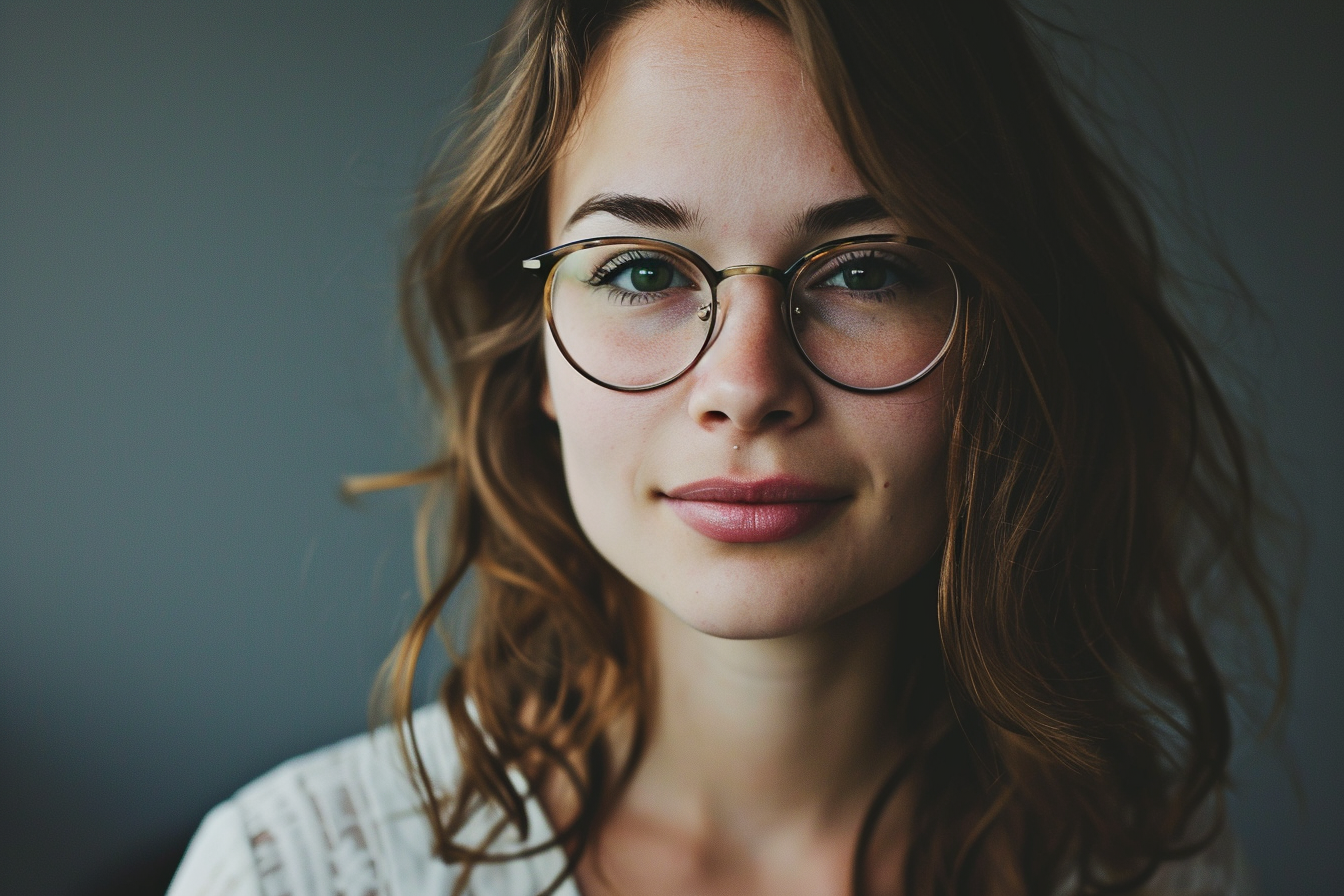