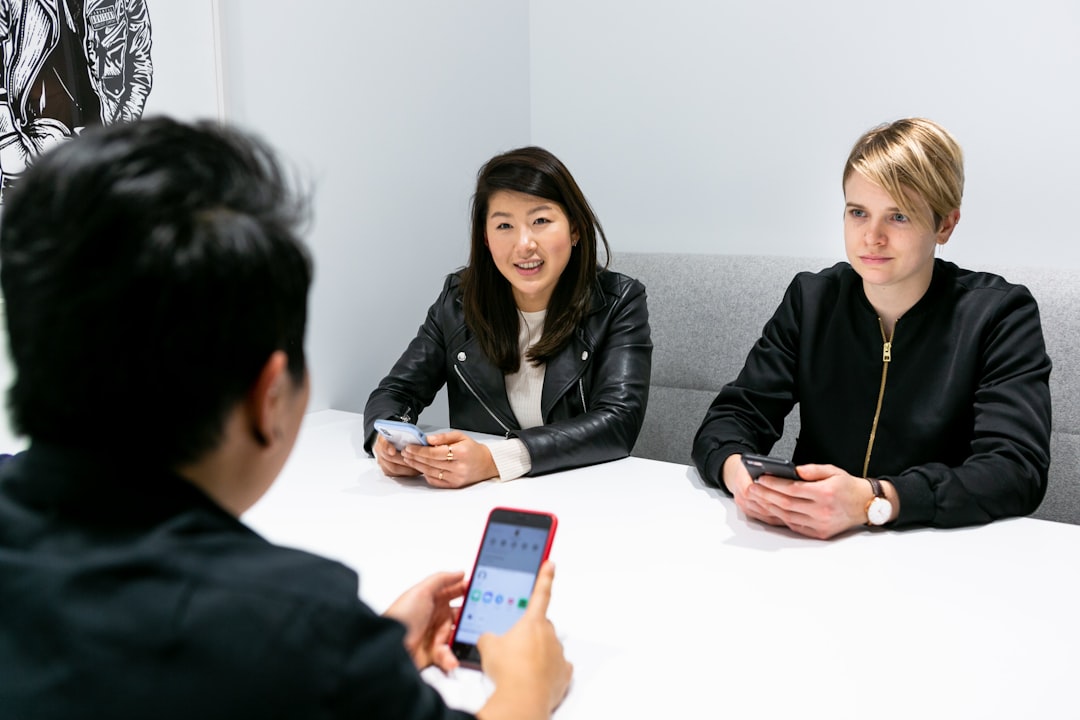
React is a JavaScript library that was developed by Facebook in 2013. It quickly gained popularity among web developers due to its simplicity and efficiency in building user interfaces. React allows developers to create reusable UI components, making it easier to manage and update complex web applications.
One of the main advantages of using React in web development is its virtual DOM (Document Object Model) implementation. The virtual DOM is a lightweight copy of the actual DOM, which allows React to efficiently update only the necessary parts of the UI when changes occur. This results in faster rendering and improved performance compared to traditional JavaScript frameworks.
Many popular companies are using React in their web development projects. Facebook, Instagram, Netflix, Airbnb, and WhatsApp are just a few examples. These companies have chosen React for its ability to handle large amounts of data and provide a seamless user experience.
Key Takeaways
- React is an important tool in web development
- Components, props, and state are core concepts in React
- React allows for building interactive UIs with event handling, forms, and validation
- React Router enables navigation and routing in single-page applications
- Redux manages state and data flow in React applications
Understanding the Core Concepts of React: Components, Props, and State
Components are the building blocks of a React application. They are reusable pieces of code that encapsulate the UI logic and can be composed together to create complex user interfaces. Components can be either functional or class-based.
Props (short for properties) are used to pass data from a parent component to its child components. They are read-only and cannot be modified by the child components. Props allow for easy communication between components and enable the creation of dynamic and interactive UIs.
State is used to manage the internal data of a component. Unlike props, state can be modified by the component itself. When the state of a component changes, React automatically re-renders the component and updates the UI accordingly.
To illustrate how components, props, and state work together in React, let’s consider an example of a simple counter component. The counter component has a state variable called “count” that keeps track of the current count value. The count value is initially set to 0 and can be incremented or decremented by clicking on buttons.
“`javascript
import React, { useState } from ‘react’;
const Counter = () => {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count – 1);
};
return (
Counter: {count}
);
};
export default Counter;
“`
In this example, the count value is stored in the state using the useState hook. The increment and decrement functions modify the count value by calling the setCount function. The current count value is displayed in the UI using JSX syntax.
Building Interactive UIs with React: Event Handling, Forms, and Validation
Topic | Description |
---|---|
Event Handling | Learn how to handle events in React, such as onClick, onChange, onSubmit, etc. |
Forms | Understand how to create and manage forms in React, including controlled and uncontrolled components. |
Validation | Explore different ways to validate user input in React, such as using regular expressions, third-party libraries, or custom validation functions. |
Event handling in React is similar to event handling in traditional JavaScript. However, React uses synthetic events instead of native browser events to ensure consistent behavior across different browsers.
To handle events in React, you can use the onClick, onChange, or onSubmit attributes on HTML elements. These attributes take a callback function that will be executed when the event occurs.
Creating forms in React is straightforward. You can use the HTML form elements such as input, textarea, and select, and handle their values using state. When a user interacts with a form element, you can update the corresponding state variable to reflect the changes.
Validating user input in React can be done by adding conditional logic to check if the input meets certain criteria. You can display error messages or disable form submission until all input fields are valid.
“`javascript
import React, { useState } from ‘react’;
const LoginForm = () => {
const [username, setUsername] = useState(”);
const [password, setPassword] = useState(”);
const [error, setError] = useState(”);
const handleUsernameChange = (event) => {
setUsername(event.target.value);
};
const handlePasswordChange = (event) => {
setPassword(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
if (username === ‘admin’ && password === ‘password’) {
setError(”);
// Perform login logic
} else {
setError(‘Invalid username or password’);
}
};
return (
);
};
export default LoginForm;
“`
In this example, the LoginForm component handles the username and password input fields using state variables. The handleUsernameChange and handlePasswordChange functions update the corresponding state variables when the input values change. The handleSubmit function is called when the form is submitted, and it performs the login logic by checking if the username and password are valid.
Working with React Router: Navigation and Routing in Single-Page Applications
React Router is a popular library for handling navigation and routing in single-page applications built with React. It allows you to define routes and render different components based on the current URL.
To use React Router, you need to install it as a dependency in your project. You can then define routes using the Route component and specify the component to render for each route.
“`javascript
import React from ‘react’;
import { BrowserRouter as Router, Route, Link } from ‘react-router-dom’;
const Home = () =>
Home
;
const About = () =>
About
;
const Contact = () =>
Contact
;
const App = () => (
);
export default App;
“`
In this example, the App component defines three routes: one for the home page, one for the about page, and one for the contact page. The Link component is used to create navigation links that will update the URL and render the corresponding component.
Managing Data with Redux: State Management and Data Flow in React Applications
Redux is a state management library that can be used with React to manage the state of an application. It provides a predictable and centralized way to manage data, making it easier to debug and test complex applications.
In Redux, the state of an application is stored in a single JavaScript object called the store. The store is immutable, meaning that it cannot be directly modified. Instead, you need to dispatch actions to update the state.
Actions are plain JavaScript objects that describe what happened in the application. They are dispatched using the store’s dispatch function and are handled by reducers.
Reducers are pure functions that take the current state and an action as arguments and return a new state. They specify how the state should be updated based on the action that was dispatched.
“`javascript
import { createStore } from ‘redux’;
// Define the initial state
const initialState = {
count: 0,
};
// Define the reducer function
const reducer = (state = initialState, action) => {
switch (action.type) {
case ‘INCREMENT’:
return { …state, count: state.count + 1 };
case ‘DECREMENT’:
return { …state, count: state.count – 1 };
default:
return state;
}
};
// Create the store
const store = createStore(reducer);
// Dispatch actions to update the state
store.dispatch({ type: ‘INCREMENT’ });
store.dispatch({ type: ‘INCREMENT’ });
store.dispatch({ type: ‘DECREMENT’ });
// Get the current state
const currentState = store.getState();
console.log(currentState); // { count: 1 }
“`
In this example, we define an initial state object with a count property set to 0. We then define a reducer function that handles two types of actions: INCREMENT and DECREMENT. When an INCREMENT action is dispatched, the count property is incremented by 1. When a DECREMENT action is dispatched, the count property is decremented by 1.
We create the store using the createStore function from Redux. We can then dispatch actions using the store’s dispatch function and get the current state using the store’s getState function.
Building Dynamic and Responsive UIs with React Hooks
React Hooks were introduced in React 16.8 as a way to use state and other React features without writing class components. Hooks allow you to reuse stateful logic between components and make it easier to manage complex UIs.
There are several built-in hooks in React, such as useState, useEffect, useContext, and useRef. The useState hook allows you to add state to functional components, while the useEffect hook allows you to perform side effects, such as fetching data or subscribing to events.
“`javascript
import React, { useState, useEffect } from ‘react’;
const Timer = () => {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds((prevSeconds) => prevSeconds + 1);
}, 1000);
return () => {
clearInterval(interval);
};
}, []);
return
Seconds: {seconds}
;
};
export default Timer;
“`
In this example, the Timer component uses the useState hook to add a seconds state variable. The useEffect hook is used to start a timer that increments the seconds value every second. The useEffect hook also returns a cleanup function that clears the interval when the component is unmounted.
Testing React Applications: Unit Testing and Integration Testing Techniques
Testing is an important part of building robust and reliable React applications. There are two main types of testing in React: unit testing and integration testing.
Unit testing involves testing individual components or functions in isolation to ensure that they work correctly. You can use testing libraries such as Jest and React Testing Library to write unit tests for your React components.
Integration testing involves testing how different components or modules work together as a whole. It ensures that the different parts of your application integrate correctly and produce the expected results. You can use tools like Cypress or Selenium for integration testing in React applications.
Here’s an example of a unit test for a simple Counter component using Jest and React Testing Library:
“`javascript
import React from ‘react’;
import { render, fireEvent } from ‘@testing-library/react’;
import Counter from ‘./Counter’;
test(‘increments the count when the increment button is clicked’, () => {
const { getByText } = render(
const incrementButton = getByText(‘Increment’);
const countElement = getByText(‘Counter: 0’);
fireEvent.click(incrementButton);
expect(countElement.textContent).toBe(‘Counter: 1’);
});
“`
In this example, we render the Counter component using the render function from React Testing Library. We then use the getByText function to get references to the increment button and the count element. We simulate a click event on the increment button using the fireEvent.click function. Finally, we assert that the count element’s text content is updated correctly.
Optimizing Performance in React Applications: Best Practices and Tools
Optimizing performance is crucial for delivering a fast and responsive user experience in React applications. There are several best practices and tools that can help you optimize the performance of your React applications.
Some best practices for optimizing performance in React applications include:
– Minimizing unnecessary re-renders by using shouldComponentUpdate or React.memo.
– Using lazy loading and code splitting to load components and resources only when needed.
– Avoiding unnecessary calculations or expensive operations in render methods.
– Using memoization techniques to cache expensive function calls or calculations.
There are also several tools available for measuring and improving performance in React applications. Some popular tools include:
– React DevTools: A browser extension that allows you to inspect and debug React components, including their props, state, and rendered output.
– Lighthouse: A tool built into Google Chrome that audits web pages for performance, accessibility, and other best practices.
– Webpack Bundle Analyzer: A tool that analyzes your Webpack bundle and provides insights into its size and composition.
– Performance Timeline: A feature of the Chrome DevTools that allows you to record and analyze the performance of your web application.
Here’s an example of using React.memo to optimize the rendering of a component:
“`javascript
import React from ‘react’;
const ExpensiveComponent = ({ data }) => {
// Expensive calculations or operations here
return
;
};
export default React.memo(ExpensiveComponent);
“`
In this example, the ExpensiveComponent performs some expensive calculations or operations. By wrapping the component with React.memo, React will memoize the component and only re-render it if the props have changed. This can help improve performance by avoiding unnecessary re-renders.
Deploying React Applications: Strategies and Tools for Production Environments
Deploying a React application involves making it available to users on the internet. There are several strategies and tools that can help you deploy your React applications in production environments.
Some common strategies for deploying React applications include:
– Hosting on a static file server: You can build your React application using tools like Webpack or Create React App and deploy the resulting static files to a file server, such as Amazon S3 or Netlify.
– Deploying to a cloud platform: Cloud platforms like AWS, Google Cloud, and Microsoft Azure provide services for hosting and deploying web applications. You can use services like AWS Elastic Beanstalk, Google App Engine, or Azure App Service to deploy your React application.
– Using a content delivery network (CDN): CDNs like Cloudflare or Fastly can cache your static assets and distribute them across multiple servers worldwide, improving the performance and availability of your React application.
There are also several tools available that can help you automate the deployment process and streamline your workflow. Some popular tools include Jenkins, Ansible, Puppet, Chef, and Docker. Jenkins is a widely used open-source automation server that allows you to automate the building, testing, and deployment of your applications. Ansible is a powerful automation tool that allows you to define and manage your infrastructure as code. Puppet and Chef are configuration management tools that help you automate the deployment and management of your infrastructure. Docker is a containerization platform that allows you to package your applications and their dependencies into lightweight, portable containers, making it easier to deploy and scale your applications. These tools can greatly simplify the deployment process and improve the efficiency of your workflow.
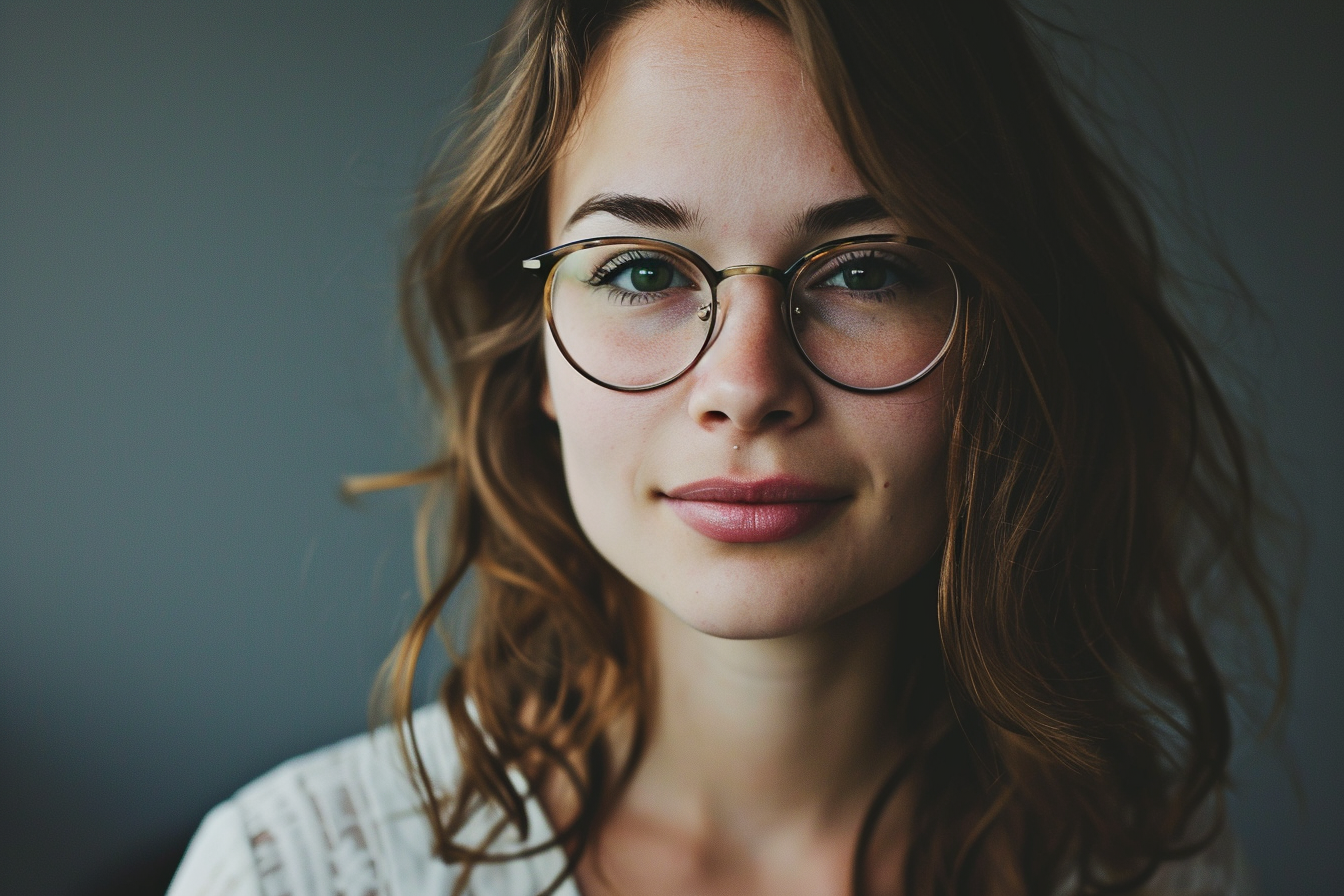