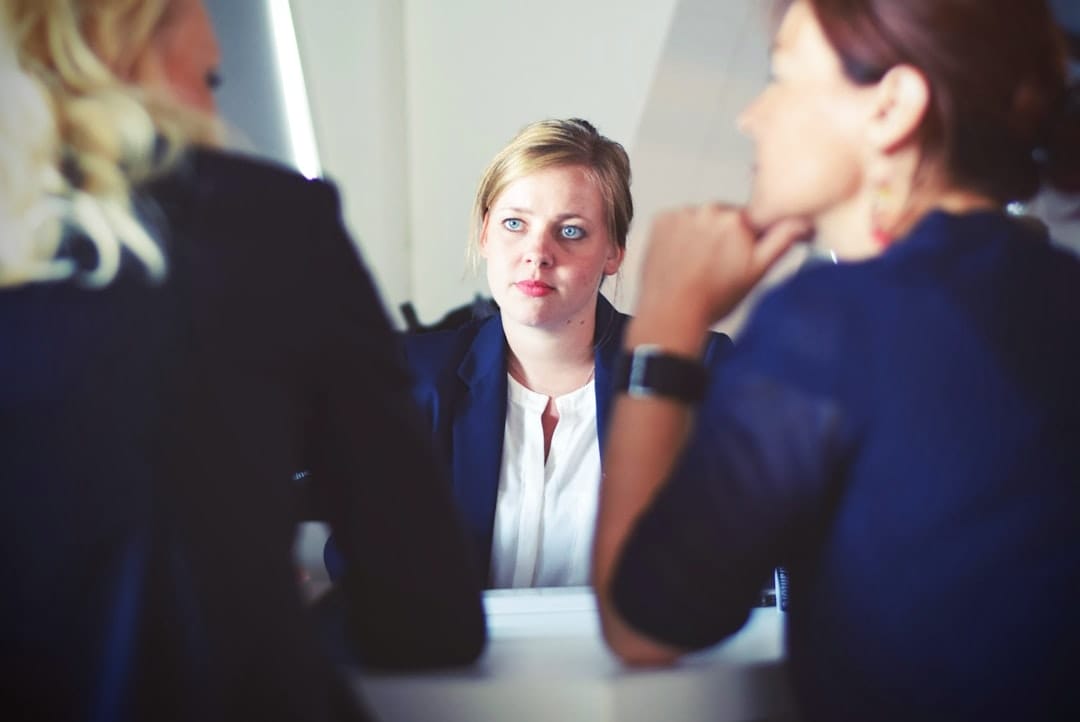
React development has become increasingly popular in recent years, with many companies and developers adopting it as their go-to framework for building user interfaces. React’s popularity can be attributed to its efficiency, flexibility, and reusability. However, in order to fully harness the power of React, it is crucial to have a solid understanding of its fundamentals. This article aims to provide a comprehensive overview of React development, covering everything from the basics to advanced concepts.
Key Takeaways
- React is a JavaScript library for building user interfaces.
- Components are the building blocks of React applications.
- State and lifecycle methods allow components to manage their own data and respond to changes.
- Hooks and Context API provide a way to share stateful logic between components.
- Debugging and testing are essential for building reliable and maintainable React applications.
Understanding the Fundamentals of React Development
React is a JavaScript library for building user interfaces. It allows developers to create reusable UI components that can be combined to build complex applications. One of the key features of React is its virtual DOM, which allows for efficient rendering and updating of components.
When compared to other frameworks like Angular or Vue.js, React stands out for its simplicity and ease of use. React’s component-based architecture makes it easy to break down complex UIs into smaller, reusable pieces. Additionally, React’s use of JSX syntax allows developers to write HTML-like code within JavaScript, making it easier to understand and maintain.
React components are the building blocks of a React application. They are reusable, self-contained pieces of code that define how a part of the user interface should look and behave. Components can be nested within each other, allowing for the creation of complex UI structures.
Props, short for properties, are used to pass data from a parent component to its child components. Props are immutable and can only be passed from parent to child. They allow for the customization and configuration of components based on their intended use.
Top Interview Questions on React Components and Props
When preparing for a React interview, it is important to have a good understanding of how components and props work in React. Here are some commonly asked interview questions on this topic:
1. What are React components and how do they work?
2. What are props in React and how are they used?
3. What is the difference between state and props in React?
4. How do you pass data from a parent component to a child component in React?
5. How do you handle events in React components?
6. What are the best practices for using components and props in React?
To answer these questions effectively, it is important to have hands-on experience with building React applications and working with components and props. It is also recommended to review the official React documentation and practice implementing different use cases.
Exploring React State and Lifecycle Methods
Topic | Description |
---|---|
State | The current data of a component that can change over time and trigger re-rendering of the component. |
Lifecycle Methods | Methods that are called at different stages of a component’s life, such as when it is mounted, updated, or unmounted. |
ComponentDidMount() | A lifecycle method that is called after a component is mounted and rendered for the first time. |
ComponentDidUpdate() | A lifecycle method that is called after a component is updated and re-rendered. |
ComponentWillUnmount() | A lifecycle method that is called before a component is unmounted and removed from the DOM. |
State is another important concept in React development. It represents the internal data of a component and can be used to store and update information that affects the rendering of the component.
Lifecycle methods in React allow developers to perform certain actions at specific points in a component’s lifecycle, such as when it is mounted or updated. These methods can be used to initialize state, fetch data from an API, or clean up resources when a component is unmounted.
When working with state and lifecycle methods, it is important to follow best practices to ensure efficient and maintainable code. Some best practices include:
1. Initialize state in the constructor method.
2. Use lifecycle methods like componentDidMount() and componentDidUpdate() to fetch data or perform side effects.
3. Avoid directly mutating state, instead use the setState() method provided by React.
4. Clean up any resources or subscriptions in the componentWillUnmount() method.
Here are some examples of using state and lifecycle methods in React:
1. Updating a counter:
“`
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
componentDidMount() {
setInterval(() => {
this.setState(prevState => ({
count: prevState.count + 1
}));
}, 1000);
}
render() {
return
;
}
}
“`
2. Fetching data from an API:
“`
class UserList extends React.Component {
constructor(props) {
super(props);
this.state = {
users: []
};
}
componentDidMount() {
fetch(‘https://api.example.com/users’)
.then(response => response.json())
.then(data => {
this.setState({
users: data
});
});
}
render() {
return (
-
- {this.state.users.map(user => (
-
- {user.name}
))}
);
}
}
“`
Mastering React Hooks and Context API
React hooks were introduced in React 16.8 as a way to write stateful functional components. They allow developers to use state and other React features without writing a class.
Some commonly used hooks include useState(), useEffect(), and useContext(). useState() allows for managing state within a functional component, useEffect() is used for performing side effects, and useContext() provides access to a context value.
The Context API in React allows for sharing data between components without having to pass props through every level of the component tree. It provides a way to create global state that can be accessed by any component within the application.
When using hooks and the Context API, it is important to follow best practices to ensure clean and maintainable code. Some best practices include:
1. Use hooks sparingly and only when necessary.
2. Keep hooks at the top level of the component.
3. Use the dependency array in useEffect() to control when the effect should run.
4. Use the useContext() hook instead of prop drilling when sharing global state.
Here are some examples of using hooks and the Context API in React:
1. Managing state with useState():
“`
import React, { useState } from ‘react’;
function Counter() {
const [count, setCount] = useState(0);
return (
Count: {count}
);
}
“`
2. Fetching data with useEffect():
“`
import React, { useState, useEffect } from ‘react’;
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch(‘https://api.example.com/users’)
.then(response => response.json())
.then(data => {
setUsers(data);
});
}, []);
return (
-
- {users.map(user => (
-
- {user.name}
))}
);
}
“`
3. Using the Context API:
“`
import React, { useContext } from ‘react’;
const UserContext = React.createContext();
function App() {
return (
);
}
function UserProfile() {
const user = useContext(UserContext);
return
Welcome, {user.name}!
;
}
“`
Tips and Tricks for Debugging React Applications
Debugging is an essential skill for any developer, and React applications are no exception. Here are some tips and tricks for debugging React applications:
1. Use console.log(): The simplest way to debug is by using console.log() statements to log values or check if certain code blocks are being executed.
2. Use the React DevTools: The React DevTools extension for Chrome and Firefox provides a powerful set of tools for inspecting and debugging React components. It allows you to view the component tree, inspect props and state, and even modify them in real-time.
3. Use the React Developer Tools in the browser: React provides a set of developer tools that can be enabled in the browser’s developer console. These tools allow you to inspect React components, view their props and state, and even simulate user interactions.
4. Use breakpoints: Set breakpoints in your code using the browser’s developer tools or a code editor like Visual Studio Code. This allows you to pause the execution of your code at a specific point and inspect the values of variables and expressions.
5. Use error boundaries: React provides a feature called error boundaries that allows you to catch and handle errors that occur within a component’s subtree. By wrapping components with an error boundary, you can prevent the entire application from crashing when an error occurs.
Best Practices for Testing React Components
Testing is an important part of the development process, as it helps ensure that your code works as expected and prevents regressions. When it comes to testing React components, there are several best practices to follow:
1. Write unit tests: Unit tests focus on testing individual components or functions in isolation. They should cover all possible use cases and edge cases to ensure that the component behaves as expected.
2. Use a testing library: There are several testing libraries available for React, such as Jest and React Testing Library. These libraries provide utilities and APIs specifically designed for testing React components.
3. Mock dependencies: When testing a component that relies on external dependencies, such as APIs or services, it is important to mock those dependencies to isolate the component being tested.
4. Test user interactions: Test how the component responds to user interactions, such as clicking buttons or entering text into input fields. Use tools like React Testing Library’s fireEvent() function to simulate user interactions.
5. Test edge cases: Make sure to test edge cases and boundary conditions, such as empty arrays or null values, to ensure that the component handles them correctly.
Here is an example of testing a React component using Jest and React Testing Library:
“`
import React from ‘react’;
import { render, fireEvent } from ‘@testing-library/react’;
import Button from ‘./Button’;
test(‘Button renders correctly’, () => {
const { getByText } = render();
const button = getByText(‘Click me’);
expect(button).toBeInTheDocument();
});
test(‘Button calls onClick handler when clicked’, () => {
const handleClick = jest.fn();
const { getByText } = render();
const button = getByText(‘Click me’);
fireEvent.click(button);
expect(handleClick).toHaveBeenCalledTimes(1);
});
“`
Optimizing React Performance: Tips and Techniques
Optimizing performance is crucial for delivering a smooth and responsive user experience. Here are some tips and techniques for optimizing React performance:
1. Use the React Profiler: The React Profiler is a built-in tool that allows you to measure the performance of your React components. It provides insights into how long each component takes to render and update, helping you identify performance bottlenecks.
2. Use memoization: Memoization is a technique that allows you to cache the results of expensive function calls and reuse them when the inputs haven’t changed. In React, you can use the useMemo() hook to memoize the value of a computed property or the useCallback() hook to memoize a callback function.
3. Avoid unnecessary re-renders: React’s reconciliation algorithm compares the previous and current versions of a component’s virtual DOM tree to determine what needs to be updated. To avoid unnecessary re-renders, make sure to use the shouldComponentUpdate() lifecycle method or the PureComponent class to perform shallow comparisons of props and state.
4. Use lazy loading and code splitting: Lazy loading and code splitting allow you to load only the necessary parts of your application when they are needed. This can significantly improve the initial load time and reduce the amount of JavaScript that needs to be downloaded.
5. Optimize rendering with React.memo(): React.memo() is a higher-order component that can be used to memoize the rendering of a functional component. It prevents unnecessary re-renders by comparing the previous and current props.
Here is an example of using React.memo() to optimize rendering:
“`
import React from ‘react’;
const MyComponent = React.memo(({ prop1, prop2 }) => {
// Component logic here
});
export default MyComponent;
“`
Advanced React Concepts: Redux and GraphQL
Redux is a state management library for JavaScript applications, and it is commonly used with React. It provides a predictable state container that allows you to manage the state of your application in a centralized manner.
GraphQL is a query language for APIs and a runtime for executing those queries with your existing data. It allows you to define the structure of the data you need, and the server responds with exactly that data.
When using Redux with React, it is important to follow best practices to ensure clean and maintainable code. Some best practices include:
1. Use Redux only when necessary: Redux introduces additional complexity to your application, so it should only be used when you have complex state management needs.
2. Use Redux Toolkit: Redux Toolkit is the recommended way to use Redux in modern applications. It provides a set of utilities and abstractions that simplify common Redux use cases.
3. Use selectors: Selectors are functions that compute derived data from the Redux store. They allow you to encapsulate complex logic and provide a consistent interface for accessing data.
When using GraphQL with React, it is important to follow best practices to ensure efficient and maintainable code. Some best practices include:
1. Use Apollo Client: Apollo Client is a powerful GraphQL client that provides a seamless integration with React. It handles caching, state management, and network requests, allowing you to focus on writing your UI components.
2. Use GraphQL fragments: Fragments allow you to define reusable pieces of a GraphQL query. They can be used to encapsulate common fields and reduce duplication in your queries.
3. Use GraphQL subscriptions: Subscriptions allow you to receive real-time updates from the server. They are useful for implementing features like live chat or real-time notifications.
Building Scalable React Applications: Architecture and Design Patterns
Building scalable React applications requires careful consideration of the application’s architecture and design patterns. Here are some best practices for building scalable React applications:
1. Use a modular architecture: Divide your application into small, reusable modules that can be developed and tested independently. This allows for easier maintenance and scalability.
2. Follow the single responsibility principle: Each component or module should have a single responsibility and should not be responsible for too many things. This makes it easier to understand, test, and maintain the code.
3. Use container and presentational components: Container components are responsible for fetching data and managing state, while presentational components are responsible for rendering the UI based on the props they receive. This separation of concerns makes it easier to reason about the code and promotes reusability.
4. Use a state management library: As your application grows, managing state becomes more complex. Using a state management library like Redux or MobX can help centralize and organize your application’s state.
5 The fifth step in the process is to evaluate the results. After implementing the solution or making the necessary changes, it is important to assess whether the desired outcome has been achieved. This involves analyzing data, gathering feedback, and comparing the current situation with the initial problem or goal. By evaluating the results, it becomes possible to determine the effectiveness of the solution and identify any areas for improvement. This step is crucial in ensuring that the problem-solving process is successful and that future decisions can be made based on accurate information.
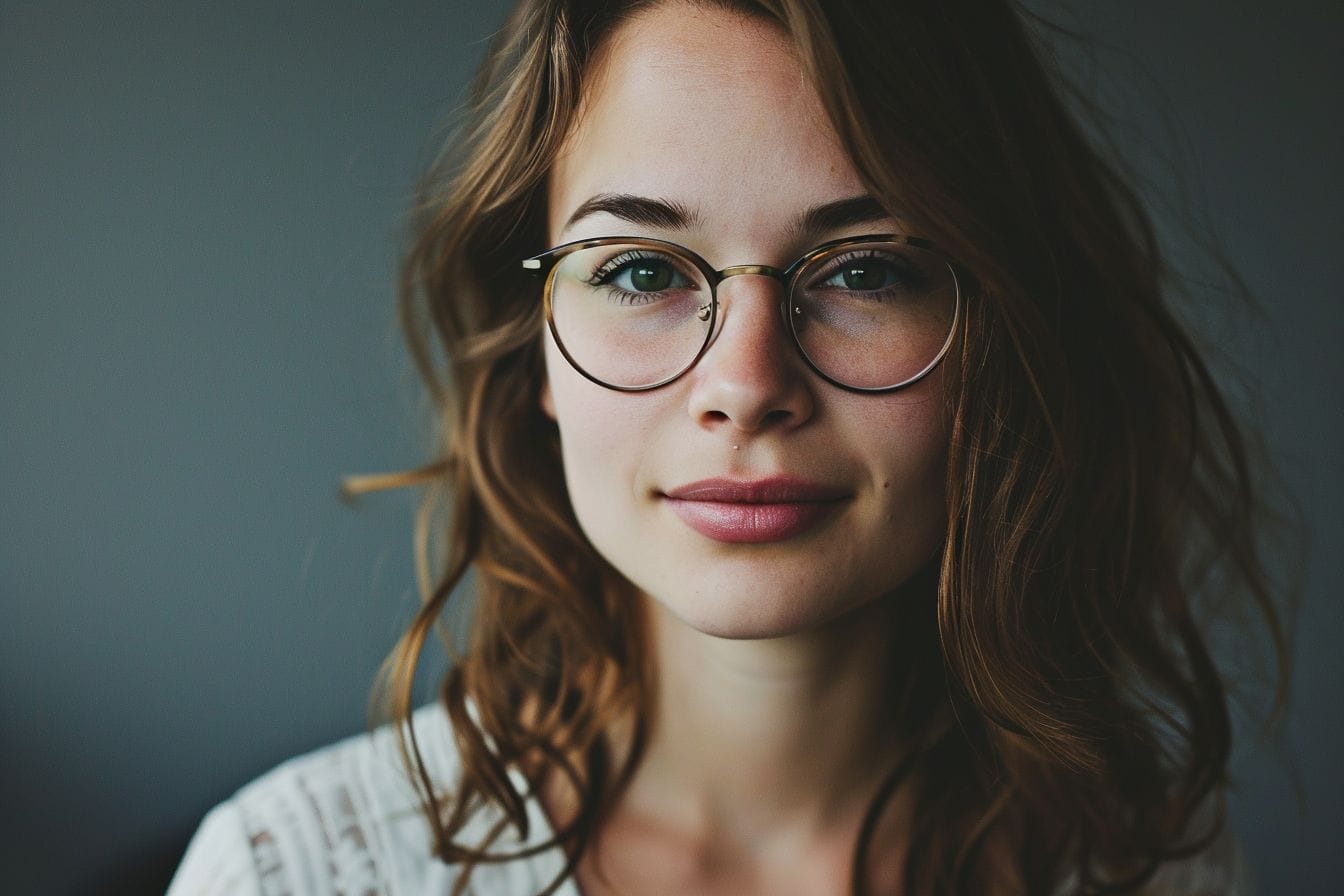